透過網頁讀取PSD後,依圖層輸出PNG檔案
在前面介紹過網頁可以讀取PSD檔案並擷取到該檔案的資訊,接著我們會希望能透過網頁上傳PSD檔之後,依照圖層來輸出圖片,同樣是利用PSD.js來輔助進行,可以透過我們製作的範例頁面來進行輸出測試。
預設輸出效果
透過PSD.js可將PSD內的各圖層輸出成PNG圖檔,亦可轉成Base 64圖檔格式,惟輸出時每個圖檔的尺寸並不會根據整體PSD檔大小來配置。
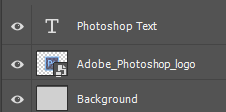

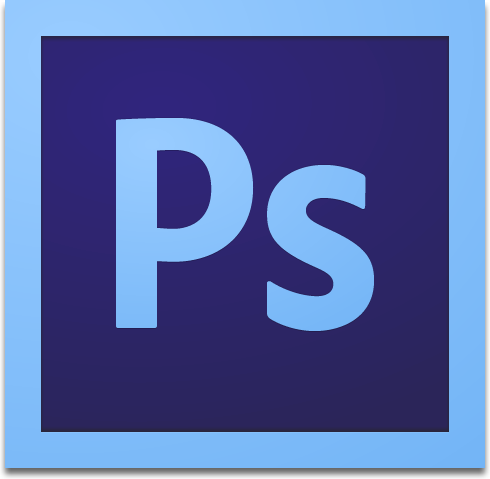
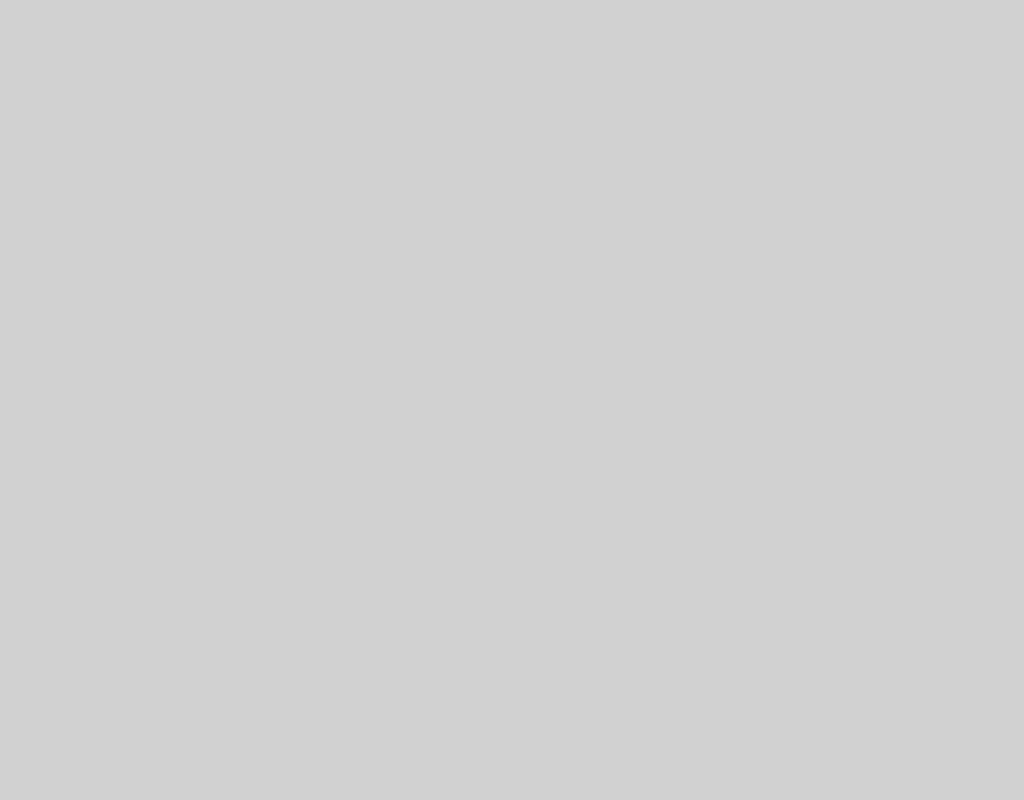
<!DOCTYPE html> <html> <head> <title>psd.js image example</title> <style type="text/css"> body, html { padding: 0; margin: 0; } #dropzone { width: 500px; height: 100px; border: 1px #ababab dashed; margin: 50px auto; } #dropzone p { text-align: center; line-height: 100px; margin: 0; padding: 0; } #image { text-align: center; } </style> <script type="text/javascript" src="psd.min.js"></script> </head> <body> <div id="dropzone"> <p>Drop PSD here</p> </div> <div id="image"></div> <pre id="data"></pre> <script type="text/javascript"> (function () { const PSD = require('psd'); document.getElementById('dropzone').addEventListener('dragover', onDragOver, true); document.getElementById('dropzone').addEventListener('drop', onDrop, true); function onDragOver(e) { e.stopPropagation(); e.preventDefault(); e.dataTransfer.dropEffect = 'copy'; } function onDrop(e) { e.stopPropagation(); e.preventDefault(); PSD.fromEvent(e).then(function (psd) { for (var i = 0; i < psd.layers.length; i ++){ document.getElementById('image').appendChild(psd.layers[i].image.toPng()); } }); } }()); </script> </body> </html>
依照整體PSD配置進行輸出
我們希望讓每個圖片在輸出後能保時相同的尺寸,也就是依照PSD的畫布大小來輸出每一張圖檔,但在原生PSD.js中並不具備這樣的功能,於是我們透過下述的方式來達成:
- 擷取PSD資訊並將圖檔轉為Base 64格式
- 產生與PSD尺寸大小相同的HTML Canvas
- 將產生的Base 64圖檔,依照原始位置放入Canvas中
- 將Canvas轉成PNG圖檔
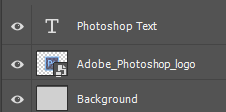
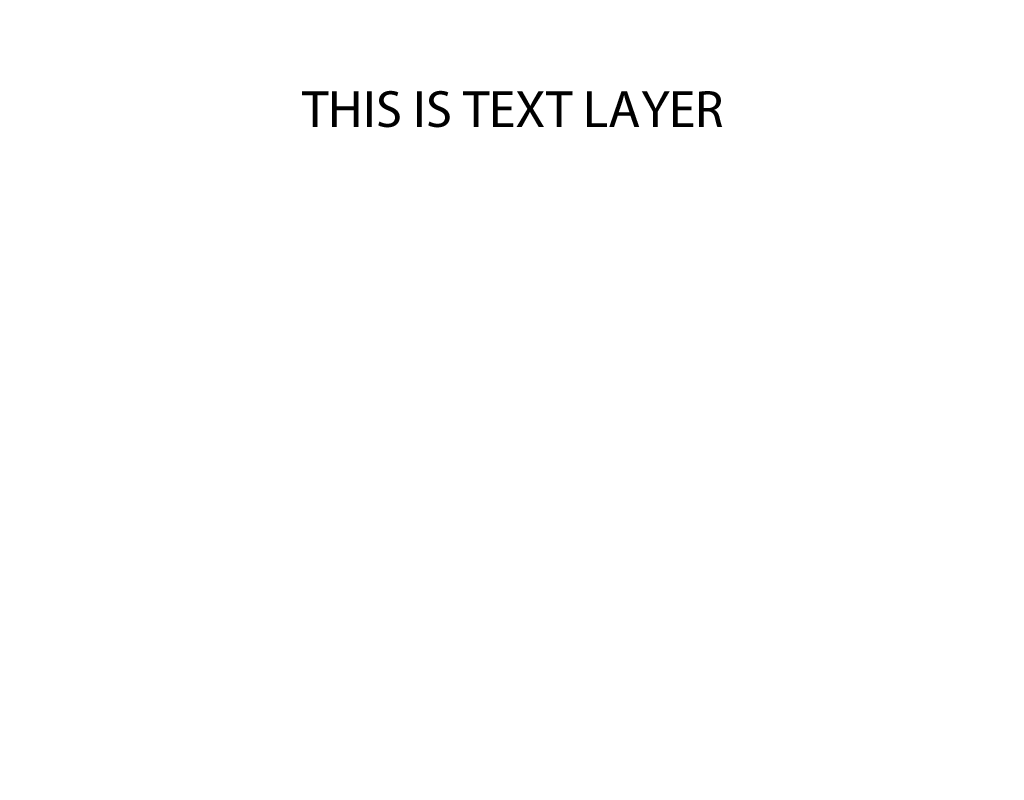
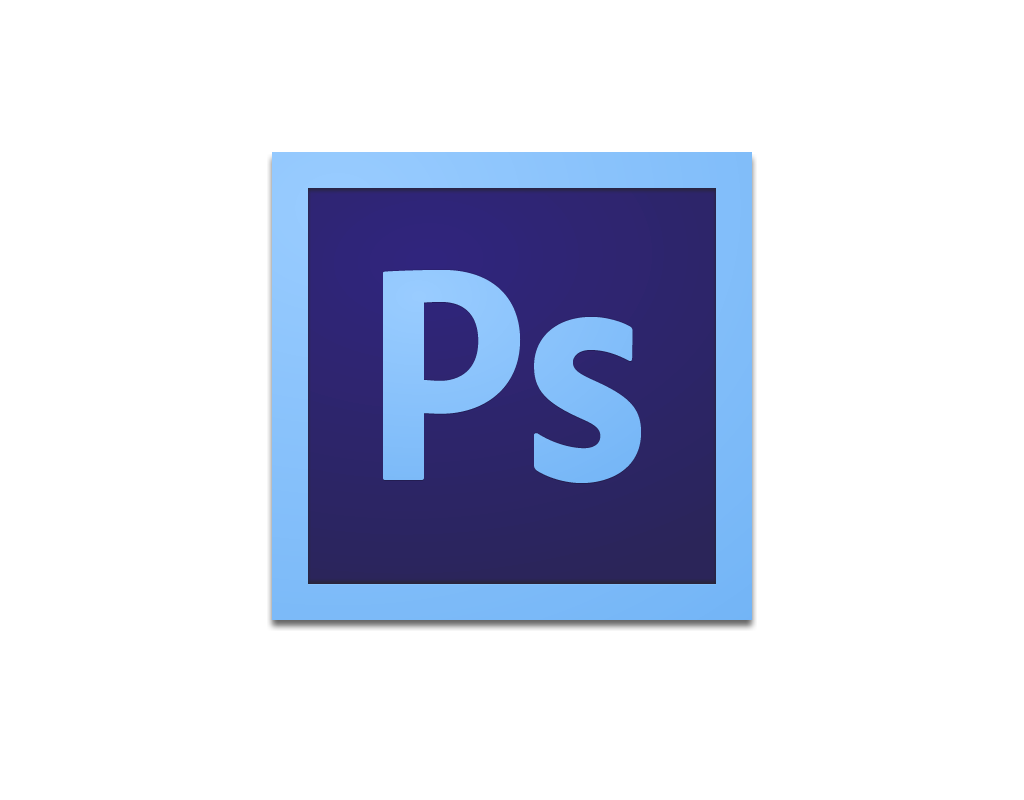
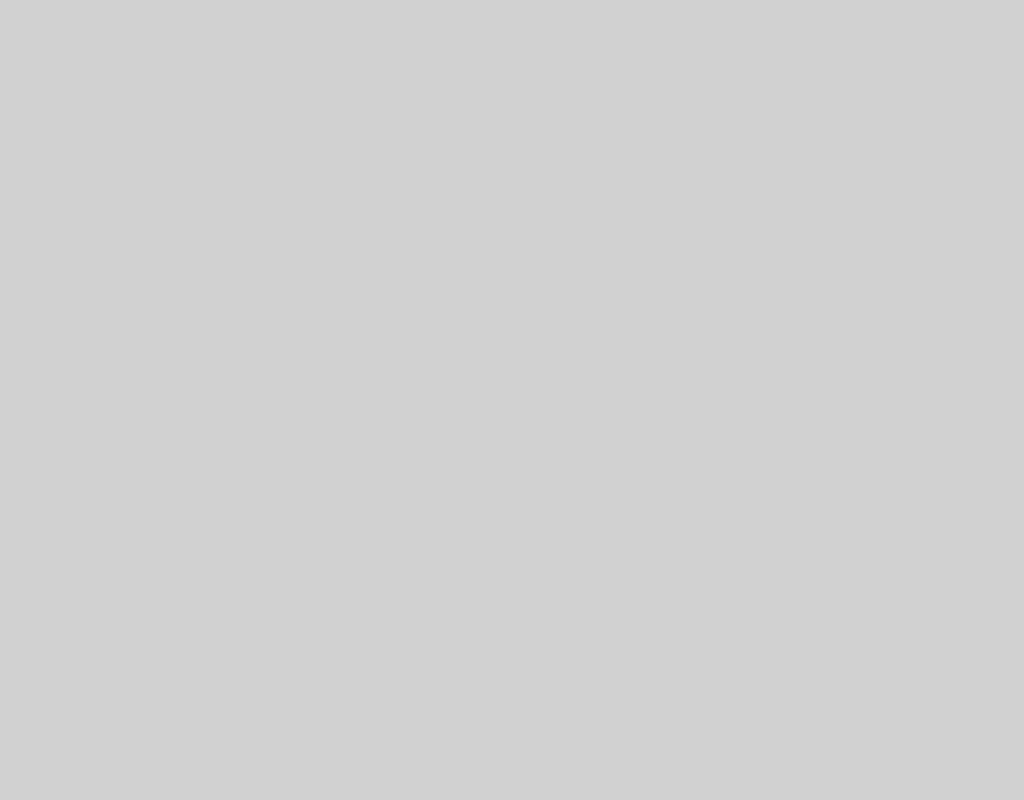
可以參考我們製作的範例頁面
<!DOCTYPE html> <html> <head> <title>psd.js image example</title> <style type="text/css"> body, html { padding: 0; margin: 0; } #dropzone { width: 500px; height: 100px; border: 1px #ababab dashed; margin: 50px auto; } #dropzone p { text-align: center; line-height: 100px; margin: 0; padding: 0; } #image { text-align: center; } </style> <script type="text/javascript" src="psd.min.js"></script> </head> <body> <div id="dropzone"> <p>Drop PSD here</p> </div> <div id="image"></div> <pre id="data"></pre> <script type="text/javascript"> (function () { const PSD = require('psd'); document.getElementById('dropzone').addEventListener('dragover', onDragOver, true); document.getElementById('dropzone').addEventListener('drop', onDrop, true); function onDragOver(e) { e.stopPropagation(); e.preventDefault(); e.dataTransfer.dropEffect = 'copy'; } function onDrop(e) { e.stopPropagation(); e.preventDefault(); PSD.fromEvent(e).then(function (psd) { const PSDWidth = psd.tree().width; const PSDHeight = psd.tree().height; for (var i = 0; i < psd.layers.length; i ++){ const img = new Image(); img.src = psd.layers[i].image.toBase64(); console.log(psd); const layerWidth = psd.layers[i].width; const layerHeight = psd.layers[i].height; const layerLeft = psd.layers[i].left; const layerTop = psd.layers[i].top; const canvas = document.createElement("canvas"); canvas.setAttribute('class', "canvas"); canvas.width = PSDWidth; canvas.height = PSDHeight; console.log("canvas :", canvas); img.onload = function(){ canvas.getContext("2d").drawImage(img, layerLeft, layerTop, layerWidth, layerHeight); document.getElementById('image').appendChild(canvas); } } }); } }()); </script> </body> </html>
PSD檔案輸出限制
1. 無法輸出帶有效果的圖片
在PSD中我們可能會針對圖層套用一些效果,例如:陰影、光暈、筆畫…等等,但在輸出後的圖檔將不會帶有這些效果。
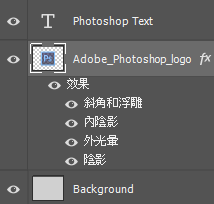
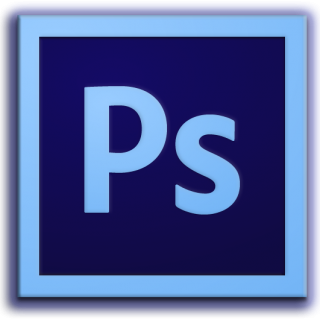
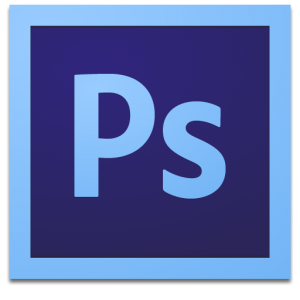
解決方法:透過點陣化圖層效果,即可輸出相對應的圖檔。
2. 無法輸出帶有遮色片效果的圖片
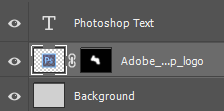
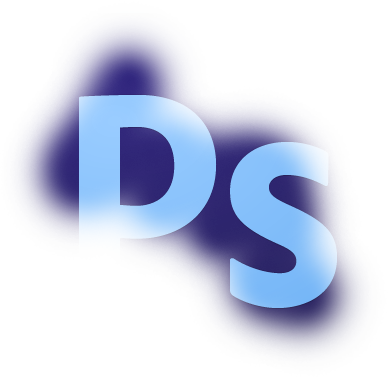
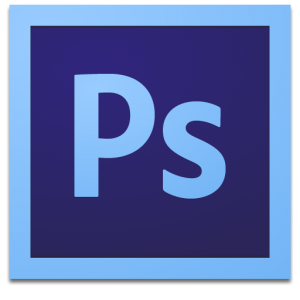
解決方法:將圖層轉為智慧型物件,即可輸出相對應的圖檔。
3. 無法依圖層混合模式輸出圖片
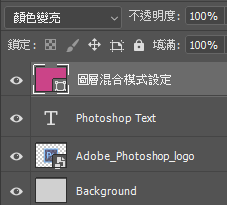
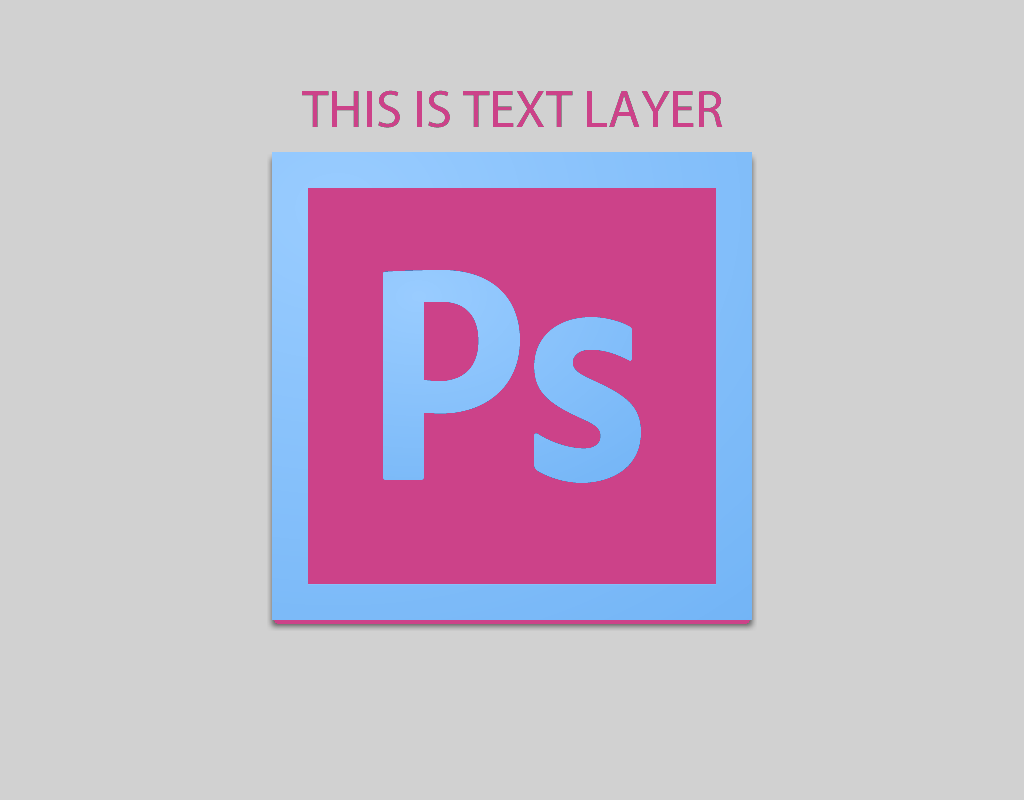
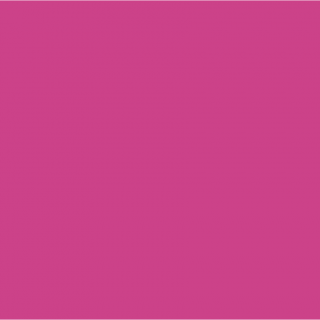

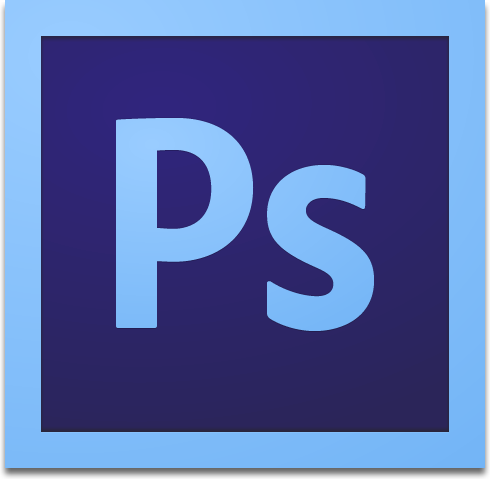
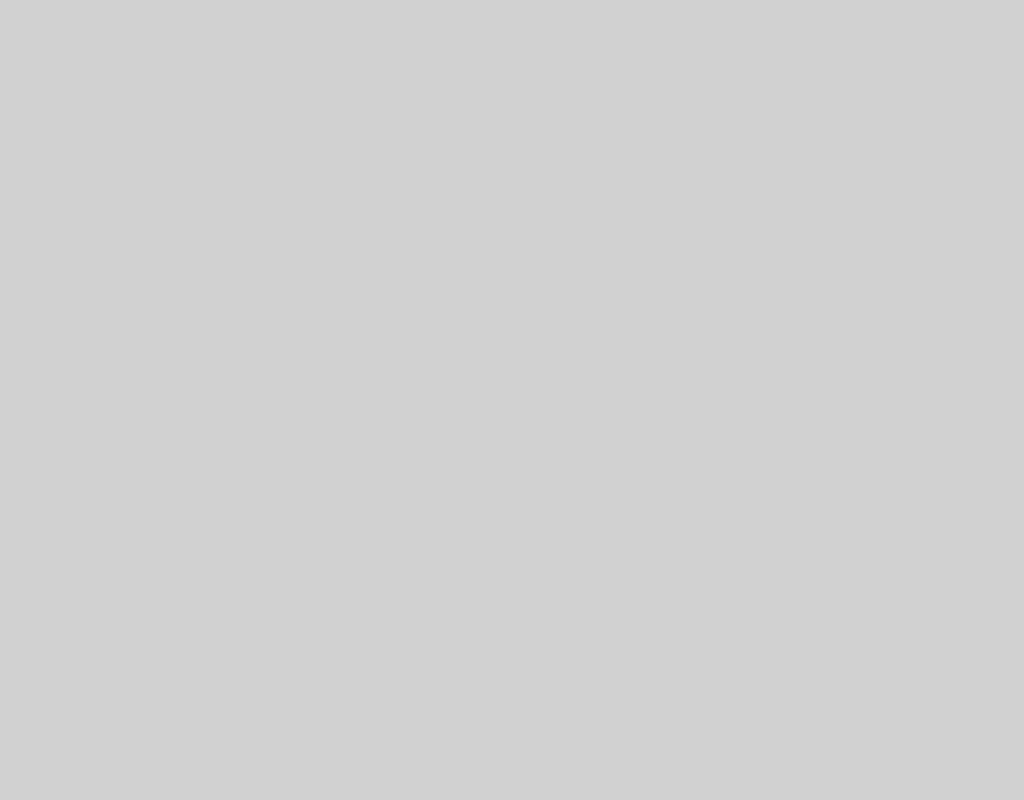
解決方法:無法解決
I would like to thank you for the efforts you have put in writing this blog. I am hoping the same high-grade blog post from you in the upcoming also. In fact your creative writing skills has encouraged me to get my own web site now. Really the blogging is spreading its wings quickly. Your write up is a good example of it.
penis enlargement
penis enlargement
пансионат мюссера веб камера стоимость путевки в сочи на 7 дней
расписание автобусов горный воздух красная талка геленджик официальный сайт цены россия алушта отзывы
крым ривьера кардиология сочи купить москвич ода
augmentin pill bactrim 960mg brand sulfamethoxazole oral
Have you ever considered creating an e-book or
guest authoring on other sites? I have a blog based on the same ideas you discuss and would
really like to have you share some stories/information. I know my readers would enjoy
your work. If you’re even remotely interested,
feel free to shoot me an e-mail.
my web page :: สาระน่ารู้ทั่วไป
I have been exploring for a little bit for any high quality articles or weblog posts in this sort of area .
Exploring in Yahoo I ultimately stumbled upon this website.
Reading this information So i’m happy to express that
I’ve an incredibly just right uncanny feeling I found out just what I needed.
I so much indisputably will make sure to do not forget this website and provides it a look regularly.
augmentin 625mg uk clavulanate brand buy sulfamethoxazole
On this site buy pouches all known PURE ICE FRESH MINT very good and trust taste!
Отличный хирург! сойфер владимир валерьевич отзывы рекомендую зайти на сайт и ознакомиться с отзывами!
cost clavulanate buy clavulanate generic bactrim brand
penis enlargement
essay writer service review
need help with essay
professional essay help
get lyrica pill can you buy cheap pregabalin tablets cost of cheap lyrica
buy viagra online
дешевые санатории россии крым ялта отели цены
гостиницы метро котельники отели в зеленоградске калининградской санаторий озера в московской области
кисловодск пикет сосновый бор татарстан санаторий официальный сайт бо прибой саки
I’m not sure why but this blog is loading
very slow for me. Is anyone else having this problem or is it a problem on my end?
I’ll check back later on and see if the problem still exists.
payday loan
What’s up, after reading this awesome article i am also glad
to share my knowledge here with colleagues.
buy viagra online
penis enlargement
I am forever thought about this, regards for putting up.
buy viagra online
отель крестовский остров санкт петербург санатория сибирь
самара гостиница ибис санатории в евпатории цены на 2021 голден тулип сочи
алладин оренбург крым санаторий курпаты отели в абхазии все включено
buy viagra online
I was curious if you ever considered changing the structure
of your site? Its very well written; I love what youve got to say.
But maybe you could a little more in the way of content so people could connect with it better.
Youve got an awful lot of text for only having 1 or 2 images.
Maybe you could space it out better?
buy viagra online
buy viagra online
ampicillin 250mg brand order ciprofloxacin sale metronidazole 400mg canada
payday loan