透過網頁讀取PSD後,依圖層輸出PNG檔案
在前面介紹過網頁可以讀取PSD檔案並擷取到該檔案的資訊,接著我們會希望能透過網頁上傳PSD檔之後,依照圖層來輸出圖片,同樣是利用PSD.js來輔助進行,可以透過我們製作的範例頁面來進行輸出測試。
預設輸出效果
透過PSD.js可將PSD內的各圖層輸出成PNG圖檔,亦可轉成Base 64圖檔格式,惟輸出時每個圖檔的尺寸並不會根據整體PSD檔大小來配置。
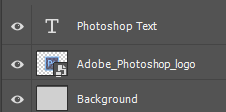

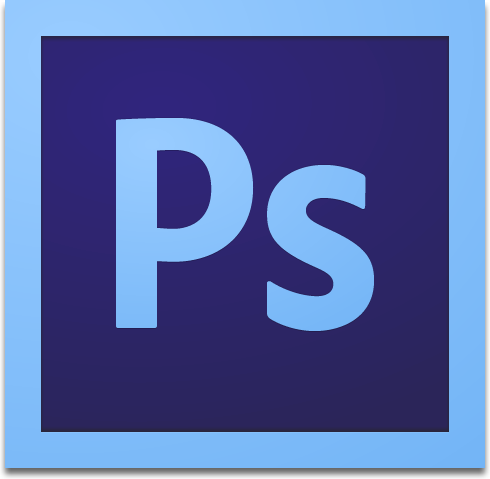
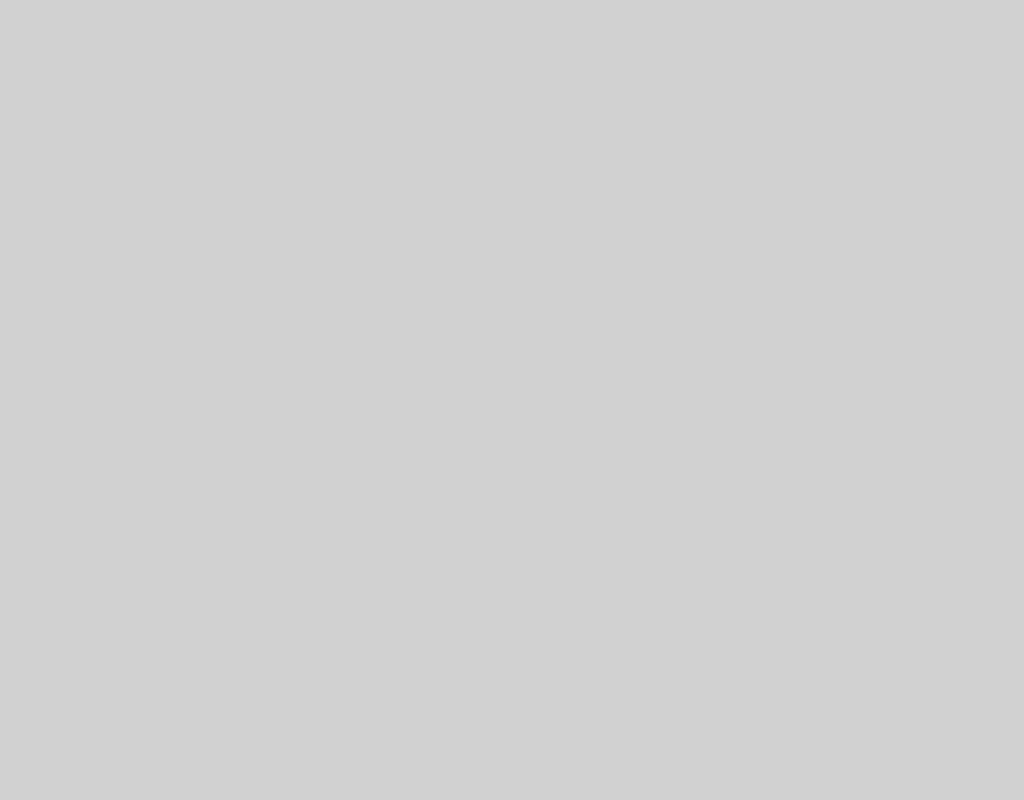
<!DOCTYPE html> <html> <head> <title>psd.js image example</title> <style type="text/css"> body, html { padding: 0; margin: 0; } #dropzone { width: 500px; height: 100px; border: 1px #ababab dashed; margin: 50px auto; } #dropzone p { text-align: center; line-height: 100px; margin: 0; padding: 0; } #image { text-align: center; } </style> <script type="text/javascript" src="psd.min.js"></script> </head> <body> <div id="dropzone"> <p>Drop PSD here</p> </div> <div id="image"></div> <pre id="data"></pre> <script type="text/javascript"> (function () { const PSD = require('psd'); document.getElementById('dropzone').addEventListener('dragover', onDragOver, true); document.getElementById('dropzone').addEventListener('drop', onDrop, true); function onDragOver(e) { e.stopPropagation(); e.preventDefault(); e.dataTransfer.dropEffect = 'copy'; } function onDrop(e) { e.stopPropagation(); e.preventDefault(); PSD.fromEvent(e).then(function (psd) { for (var i = 0; i < psd.layers.length; i ++){ document.getElementById('image').appendChild(psd.layers[i].image.toPng()); } }); } }()); </script> </body> </html>
依照整體PSD配置進行輸出
我們希望讓每個圖片在輸出後能保時相同的尺寸,也就是依照PSD的畫布大小來輸出每一張圖檔,但在原生PSD.js中並不具備這樣的功能,於是我們透過下述的方式來達成:
- 擷取PSD資訊並將圖檔轉為Base 64格式
- 產生與PSD尺寸大小相同的HTML Canvas
- 將產生的Base 64圖檔,依照原始位置放入Canvas中
- 將Canvas轉成PNG圖檔
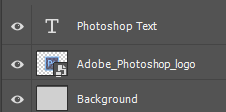
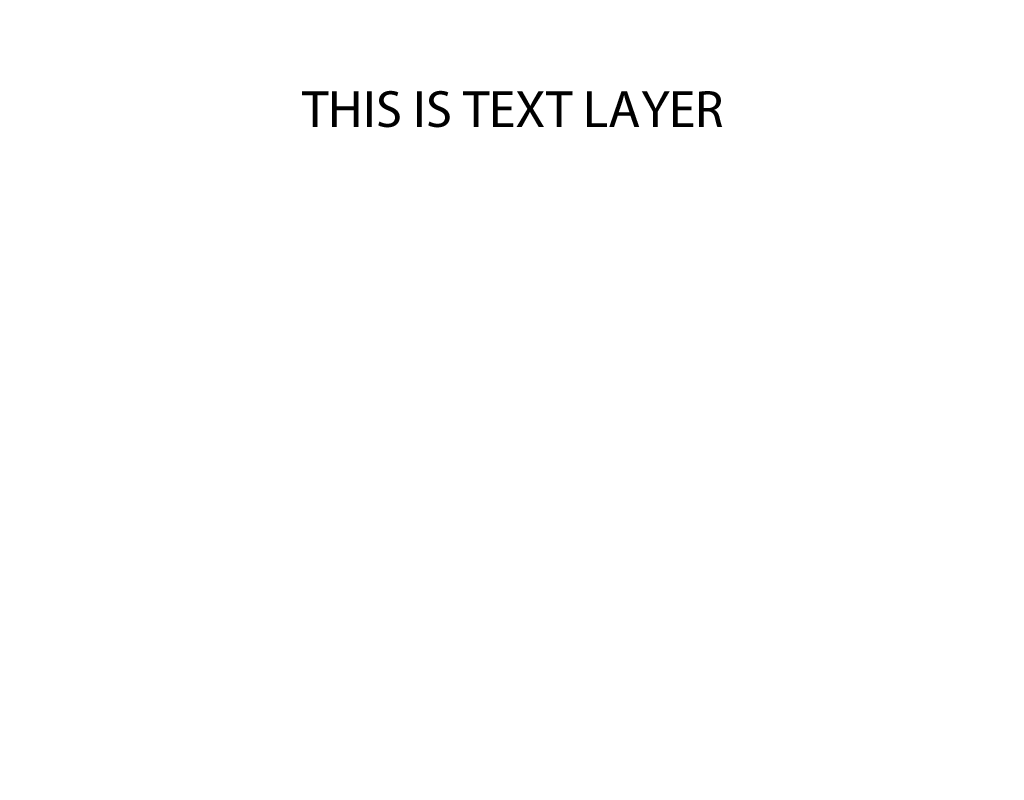
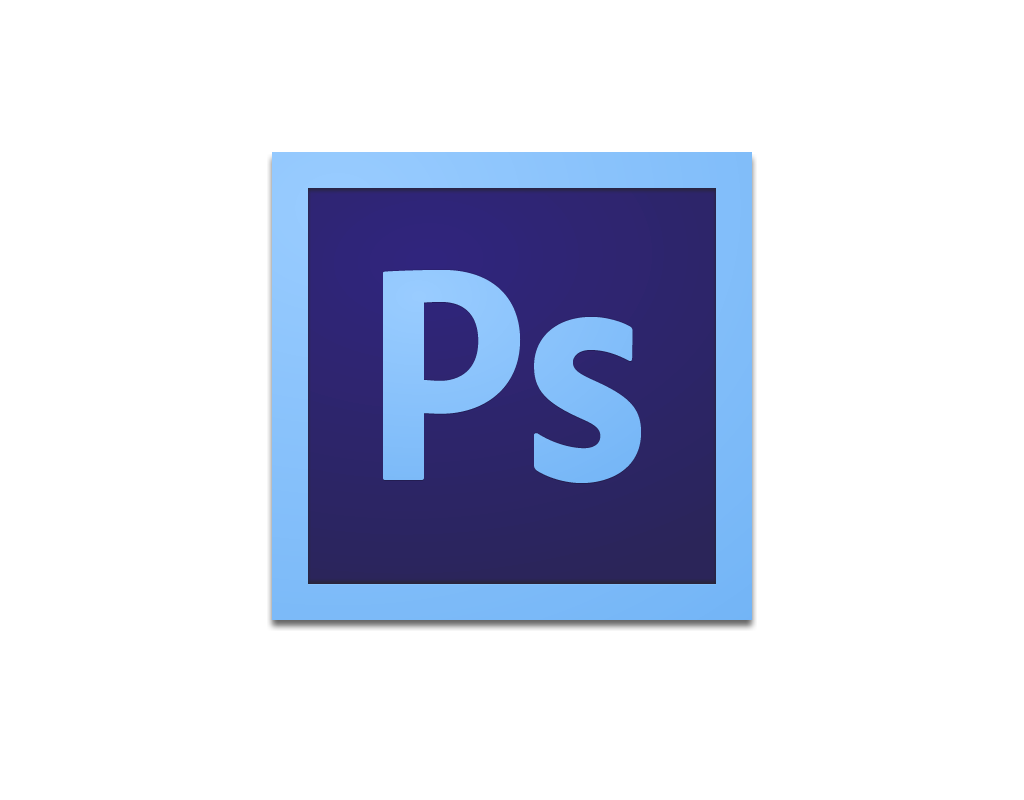
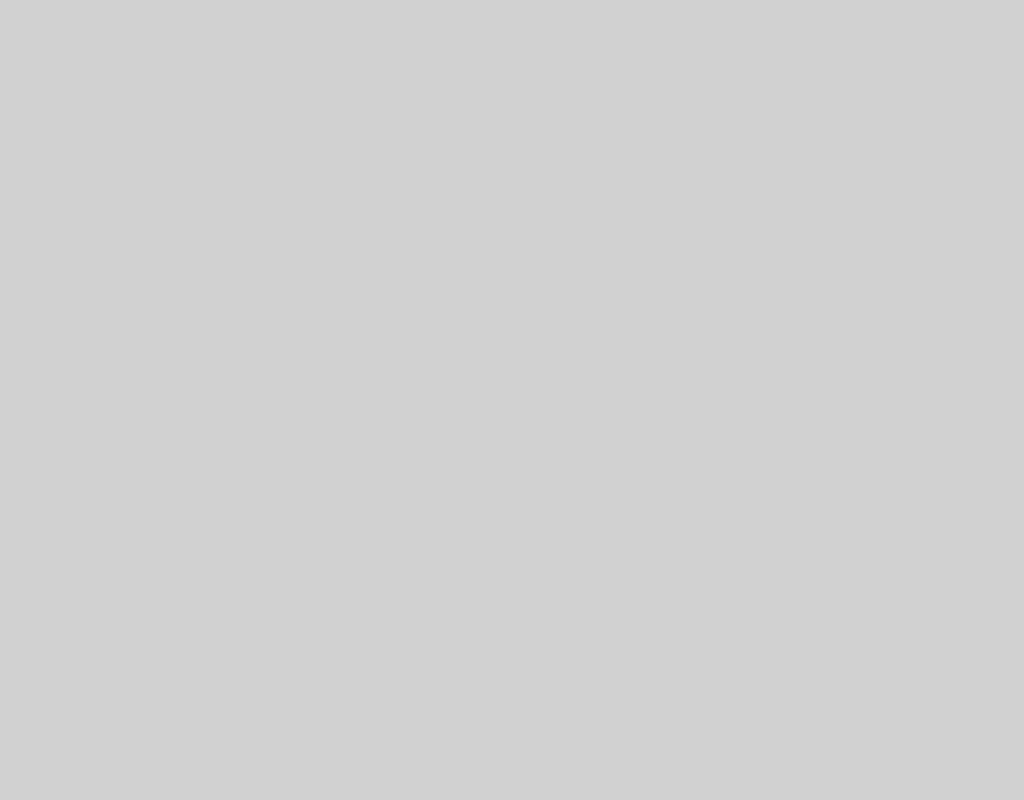
可以參考我們製作的範例頁面
<!DOCTYPE html> <html> <head> <title>psd.js image example</title> <style type="text/css"> body, html { padding: 0; margin: 0; } #dropzone { width: 500px; height: 100px; border: 1px #ababab dashed; margin: 50px auto; } #dropzone p { text-align: center; line-height: 100px; margin: 0; padding: 0; } #image { text-align: center; } </style> <script type="text/javascript" src="psd.min.js"></script> </head> <body> <div id="dropzone"> <p>Drop PSD here</p> </div> <div id="image"></div> <pre id="data"></pre> <script type="text/javascript"> (function () { const PSD = require('psd'); document.getElementById('dropzone').addEventListener('dragover', onDragOver, true); document.getElementById('dropzone').addEventListener('drop', onDrop, true); function onDragOver(e) { e.stopPropagation(); e.preventDefault(); e.dataTransfer.dropEffect = 'copy'; } function onDrop(e) { e.stopPropagation(); e.preventDefault(); PSD.fromEvent(e).then(function (psd) { const PSDWidth = psd.tree().width; const PSDHeight = psd.tree().height; for (var i = 0; i < psd.layers.length; i ++){ const img = new Image(); img.src = psd.layers[i].image.toBase64(); console.log(psd); const layerWidth = psd.layers[i].width; const layerHeight = psd.layers[i].height; const layerLeft = psd.layers[i].left; const layerTop = psd.layers[i].top; const canvas = document.createElement("canvas"); canvas.setAttribute('class', "canvas"); canvas.width = PSDWidth; canvas.height = PSDHeight; console.log("canvas :", canvas); img.onload = function(){ canvas.getContext("2d").drawImage(img, layerLeft, layerTop, layerWidth, layerHeight); document.getElementById('image').appendChild(canvas); } } }); } }()); </script> </body> </html>
PSD檔案輸出限制
1. 無法輸出帶有效果的圖片
在PSD中我們可能會針對圖層套用一些效果,例如:陰影、光暈、筆畫…等等,但在輸出後的圖檔將不會帶有這些效果。
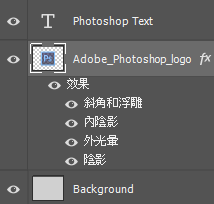
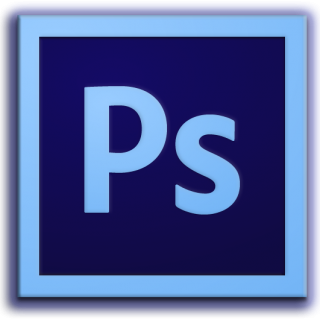
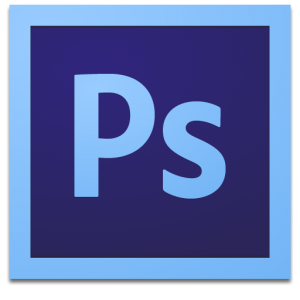
解決方法:透過點陣化圖層效果,即可輸出相對應的圖檔。
2. 無法輸出帶有遮色片效果的圖片
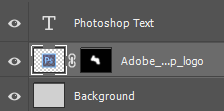
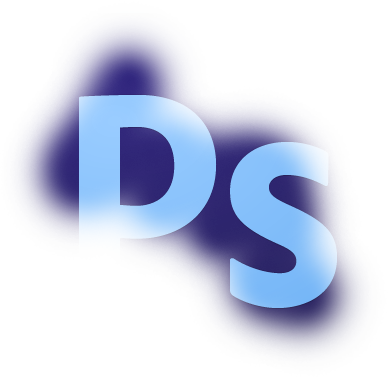
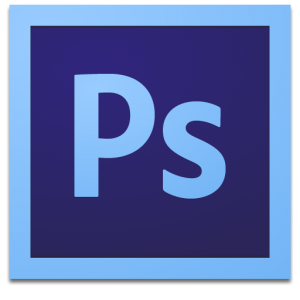
解決方法:將圖層轉為智慧型物件,即可輸出相對應的圖檔。
3. 無法依圖層混合模式輸出圖片
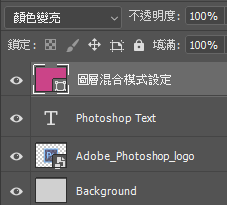
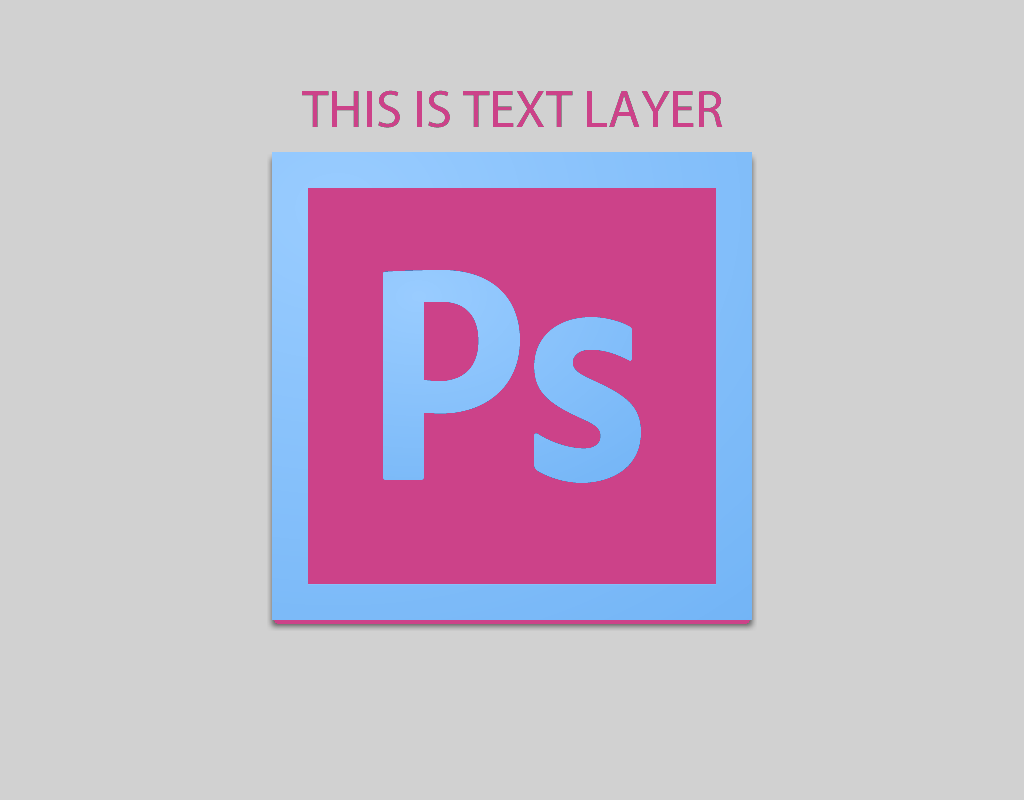
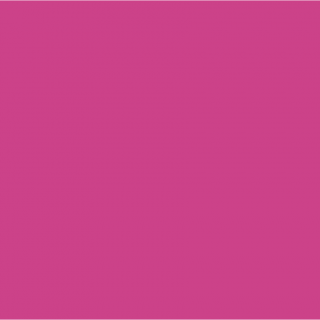

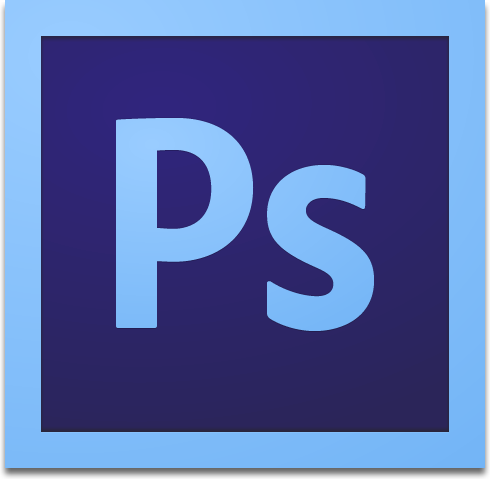
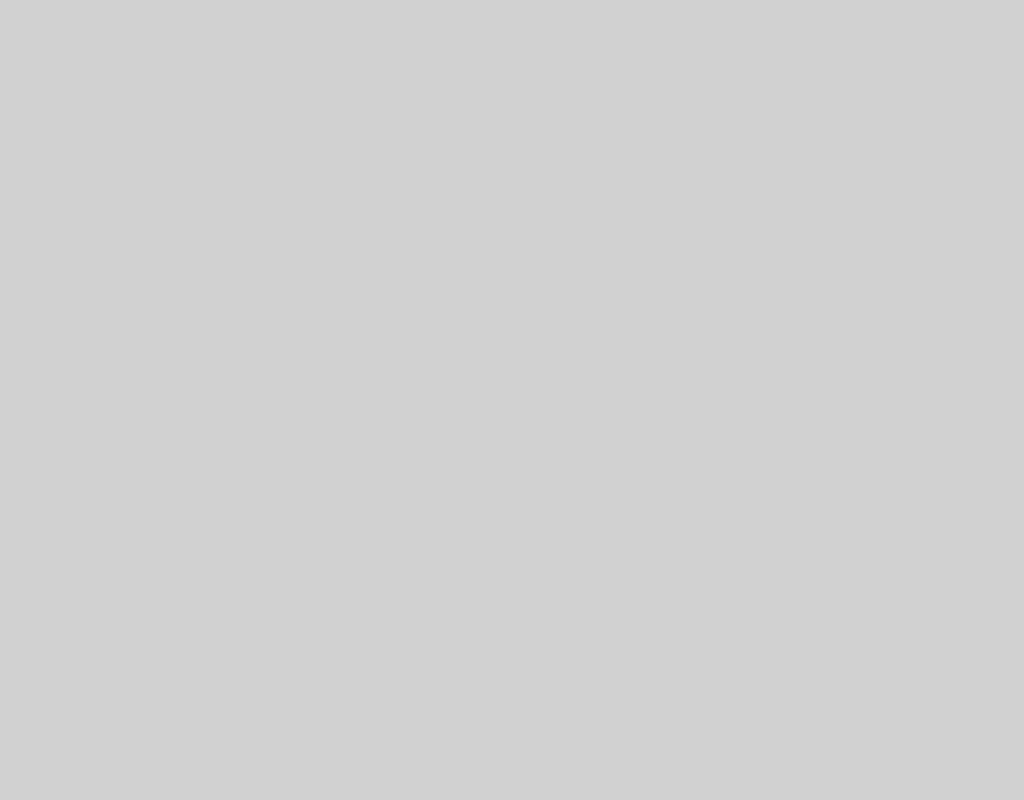
解決方法:無法解決
It’s remarkable to pay a quick visit this website and reading the views
of all friends concerning this paragraph, while I am also
eager of getting knowledge.
constantly i used to read smaller articles which also clear their motive, and that is also happening with
this article which I am reading at this place.
Way cool! Some very valid points! I appreciate you writing this post and also
the rest of the site is very good.
Hey there! Someone in my Myspace group shared this website with us so I came to look it over.
I’m definitely enjoying the information. I’m book-marking and will be tweeting this to my
followers! Excellent blog and amazing design and style.
Hi, yes this post is really good and I have learned lot of things from
it concerning blogging. thanks.
Good day! I know this is somewhat off topic but I was wondering if you knew where I could get a captcha plugin for
my comment form? I’m using the same blog platform as yours and I’m having problems finding one?
Thanks a lot!
Hi my family member! I want to say that this article is awesome, nice written and come
with almost all vital infos. I’d like to peer extra
posts like this .
Seo Always Be Invisible, However It Is Not Hidden 구글SEO (signaturesupportprogram.net)
What’s up Dear, are you truly visiting this web page on a regular basis, if so after
that you will definitely get good know-how.
Appreciating the hard work you put into your blog and detailed information you
provide. It’s nice to come across a blog every once in a while that isn’t the same out of date rehashed information. Fantastic read!
I’ve bookmarked your site and I’m including your RSS feeds to my Google account.
Hi there! Do you use Twitter? I’d like to
follow you if that would be ok. I’m undoubtedly enjoying your blog and look forward to new updates.
Hi there colleagues, fastidious paragraph and good urging commented at this place,
I am truly enjoying by these.
Have you ever considered writing an e-book or guest authoring on other blogs?
I have a blog centered on the same information you discuss and would really like to have you share some stories/information. I know my readers would appreciate your work.
If you are even remotely interested, feel free to shoot me an email.
What NOT To Do In The Anxiety Disorder Physical Symptoms Industry http://www.5097533.xyz
bookmarked!!, I really like your site!
Repairs To Upvc Windows Tools To Help You Manage Your Daily
LifeThe One Repairs To Upvc Windows Trick That Should Be Used By Everyone Know Repairs To upvc windows (telegra.ph)
We are a group of volunteers and opening a new scheme
in our community. Your web site offered us with
valuable info to work on. You’ve done an impressive job and our whole
community will be grateful to you.
Hello, i think that i noticed you visited my weblog so i came to return the desire?.I’m
trying to to find issues to improve my website!I suppose its
adequate to make use of some of your ideas!!
Hey there! This post could not be written any better!
Reading this post reminds me of my old room mate! He always kept talking about this.
I will forward this page to him. Pretty sure he will
have a good read. Many thanks for sharing!
This post will help the internet visitors for setting up new web site
or even a weblog from start to end.
Военный адвокат Запорожье. Бесплатная консультация
военный адвокат Украина
— это опытный специалист имеющий высшее юридическое образование, сдавший квалификационный государственный экзамен на право осуществления адвокатской деятельностью и специализирующийся в основном на военных делах
Вся правовая помощь военного адвоката осуществляется в индивидуальном порядке, грамотно, четко и в соответствии с действующими нормативно-правовыми актами.
Мы как военные юристы действуем не против органов Украины или министерства обороны, мы действуем во благо Украины — наших защитников и граждан Украины, которые попали в тяжелую жизненную ситуацию связанную с незнанием военного и действующего законодательства.
Поскольку, проявив патриотизм и чувство гражданской ответственности – став на защиту суверенитета страны, граждане участвующие и помогавшие в обороне после, становятся никому не нужными, особенно если военнослужащий стал инвалидом, потерял часть тела или конечность, и не может самостоятельно защитить свои права. Именно в таких ситуациях мы как военные адвокаты приходим на помощь, и добиваемся в установленном законом порядке справедливости, необходимых выплат, установление статуса, оформление пенсий, льгот и т.п.
Тоже касается, и получение отсрочки от мобилизации, когда например, безосновательно призывают сына у которого отец инвалид 2 группы, или мать прикованная из-за тяжелой болезни к постели, и требующая постороннего ухода. Это же относится и к военнослужащим, рапорта которых не регистрируются в канцелярии воинской части и полностью игнорируются, под прикрытием суеты боевых действий..
Именно в таких ситуациях, мы приходим на помощь и с помощью ЗАКОННЫХ методов правовой защиты, используя свой опыт полученный при ведении аналогичных военных дел добиваемся справедливости.
This is very interesting, You are a very skilled blogger.
I have joined your feed and look forward to
seeking more of your magnificent post. Also, I have shared your website in my social networks!
Which Keyword Sells? – Keyword Research Techniques 구글 상위노출 백링크
Excellent blog here! Also your website loads up fast! What host are you
using? Can I get your affiliate link to your host?
I wish my website loaded up as fast as yours lol
I’ve been exploring for a little bit for any high-quality articles or blog posts in this sort of
area . Exploring in Yahoo I finally stumbled upon this website.
Reading this info So i’m satisfied to show that I have an incredibly good uncanny feeling I discovered just what I needed.
I so much certainly will make certain to do not
put out of your mind this site and give it a look regularly.
After I initially left a comment I seem to have clicked on the -Notify me when new comments
are added- checkbox and from now on every time a comment is added I recieve 4 emails with the same comment.
Perhaps there is a means you are able to remove me from that service?
Many thanks!
Very good blog post. I absolutely appreciate this website.
Continue the good work!
I will immediately take hold of your rss as I can not in finding your
email subscription link or e-newsletter service. Do you have
any? Please allow me understand in order that I may just subscribe.
Thanks.
Whats up very cool site!! Guy .. Beautiful .. Superb ..
I will bookmark your site and take the feeds additionally?
I am glad to find numerous useful info here within the post,
we’d like develop extra techniques in this regard, thanks for sharing.
. . . . .
12 Companies Leading The Way In Veterans Disability Lawsuit boerne veterans disability law firm