vue.js 學習手冊 框架的選擇與導入
這篇文章是vue.js學習手冊的第一篇文章,也是我認為最難寫的一篇文章,就像vue.js提到的他是一個“漸進式”框架,在這篇文章也想要跟各位分享選擇框架的一些原則,讓大家可以“漸進式”的了解為什麼我們在網頁開發時需要選擇一個框架來幫助我們,在選擇框架之前我們要先弄清楚,框架究竟可以幫助我們在網頁開發上的哪些部分,如果這些部分跟你要開發的項目並不媒合,那奉勸你別把單純的事情搞複雜了,而且你可能會開始討厭學習框架,但若反之,你一定會愛上框架,甚至覺得他讓你事半功倍。
強大的前、後端串接功能
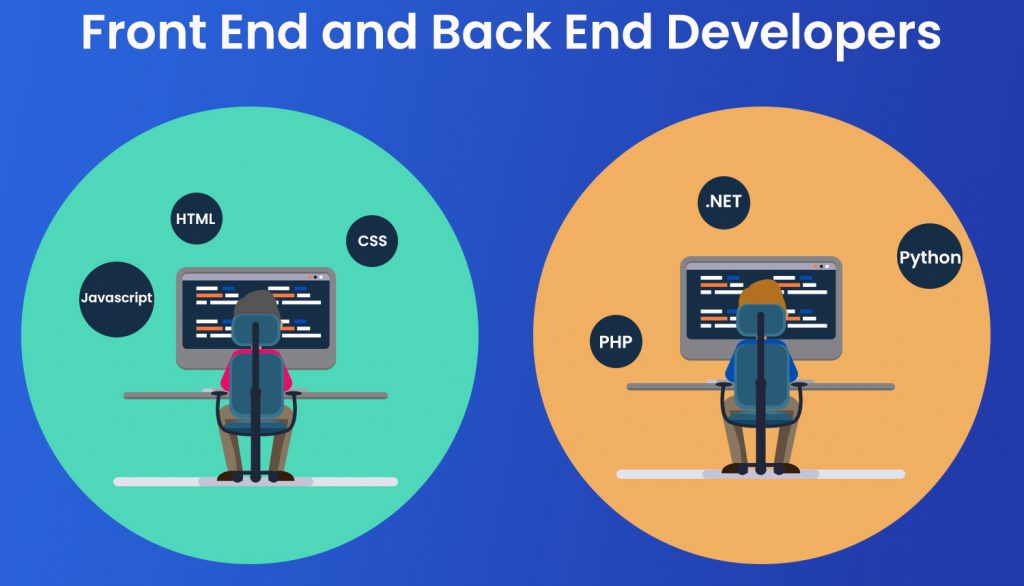
現代的網頁被要求除了有著摩登的前端UI之外,在網頁中的資料有常需要配合“大數據”下的資料進行呈現,說白話一點也就是網頁上面呈現的資料並不是寫死在頁面中的,而是透過後端資料庫取出來的,舉凡會員登入的名稱、購物網站中的商品資訊、新聞網站中的新聞就連你現在看到的這篇文章,也都是存放於資料庫中,網頁去對資料庫進行讀取後顯示在介面上的。
當然除了對資料庫進行讀取之外,網頁也會對資料庫進行儲存的動作,舉凡會員資料修改、商品訂單建立、網站偏好設定…等等,而框架在這方面有許多很好的方法,讓我們可以更周全快速的處理這方面的動作,節省許多開發的時間與減少Bug上的產生。
模組化開發架構
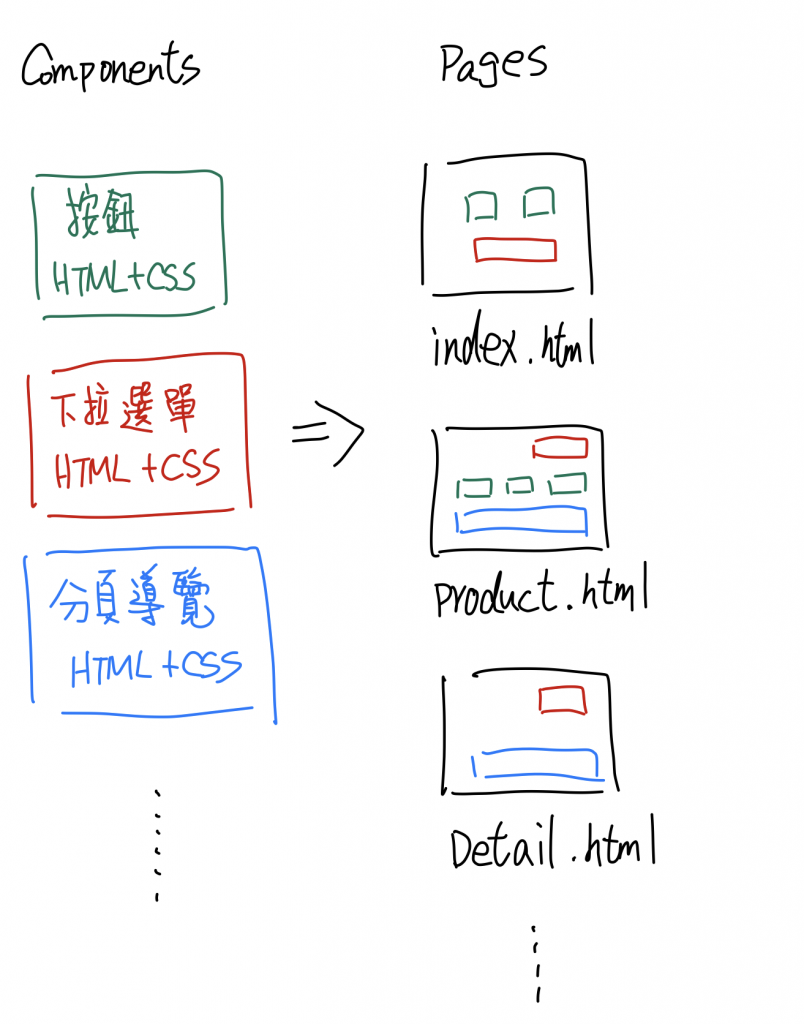
在一個大型網站中,可能有許多網頁中會出現相同風格的元素,例如:下拉式選單、按鈕、分頁導覽,是每一個頁面都會重複應用到的一些元件,傳統的網頁開發上就是在每一頁嵌入對應的HTML Code,這樣的做法非但不易維護,也會增加許多冗長且重複的程式碼。
模組化開發可以如上圖所示,將頁面中需重用的元素拉出來設計成一個Component,在不同頁面可以透過引入的方式置入該Component,而Component的維護可以統一在該Component中進行,可以減少大量維護上的時間。
透過 Virtual DOM 來提升頁面效能
現代的網頁前端框架為了提升頁面操作的效能都提供了Virtual DOM,在Vue.js 2.0中也引入Virtual DOM,比Vue.js 1.0的初始渲染速度提升了2~4倍,並大大降低了內存消耗,至於為何Virtual DOM能提昇網頁的效能,大家就必須了解我們透過Javascirpt更新實體DOM時會產生的效能問題開始了解。
實體DOM更新的效能測試
這邊製作一個簡單的範例對實體DOM和虛擬DOM的效能進行說明:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title></title> </head> <body> <div class="wrapper"> <div class="container"> <div class="itemList"> <ul id="itemList__ul"> <li id="liID">Item 1</li> </ul> </div> </div> <button onClick="insertItems()">Go</button> </div> </body> </html> <script> var itemData = ""; function insertItems() { for (var i = 1; i <= 100000; i++) { itemData = "Item " + i document.getElementById("liID").innerHTML = itemData; } } </script>
在HTML DOM的操作上,只要頁面元素有變更,就可能會觸發Reflow或Repaint這樣的動作,瀏覽器也會耗費相當多的資源在進行這些動作,以上述的例子來看,當我們按下頁面上的按鈕之後,就會透過迴圈去改變li的內容,這樣將會觸發多次的瀏覽器動作。
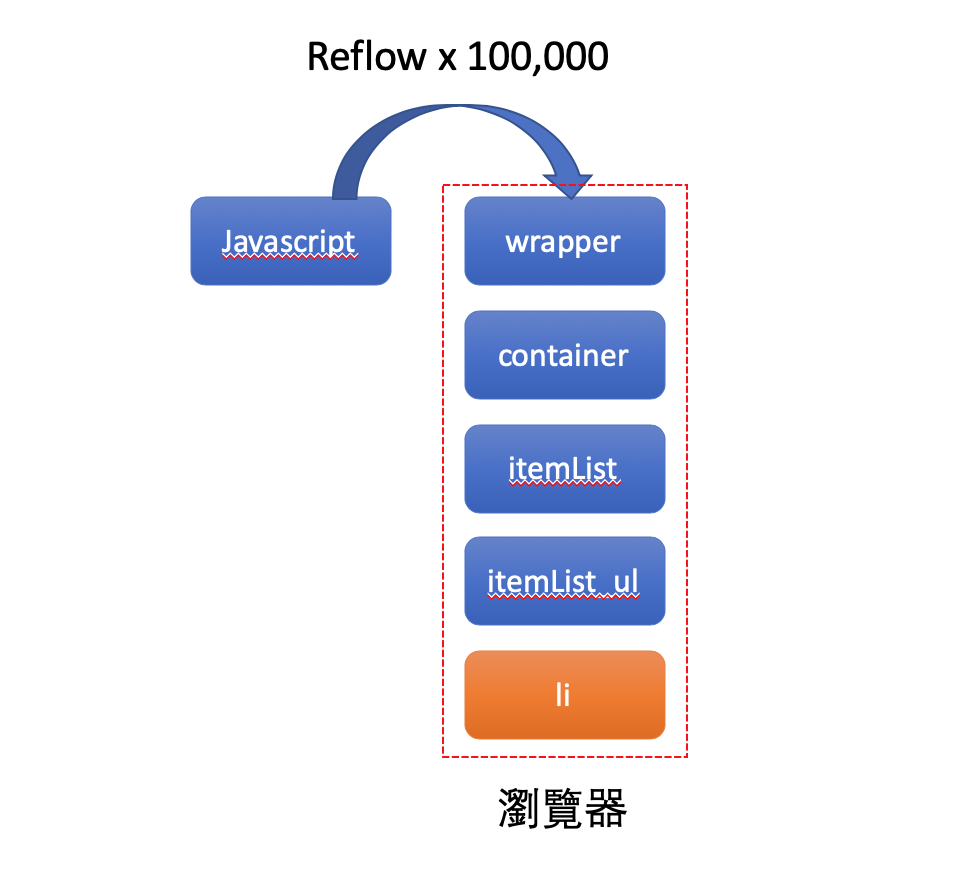
下圖是我們在Chrome中獲得的效能資訊:
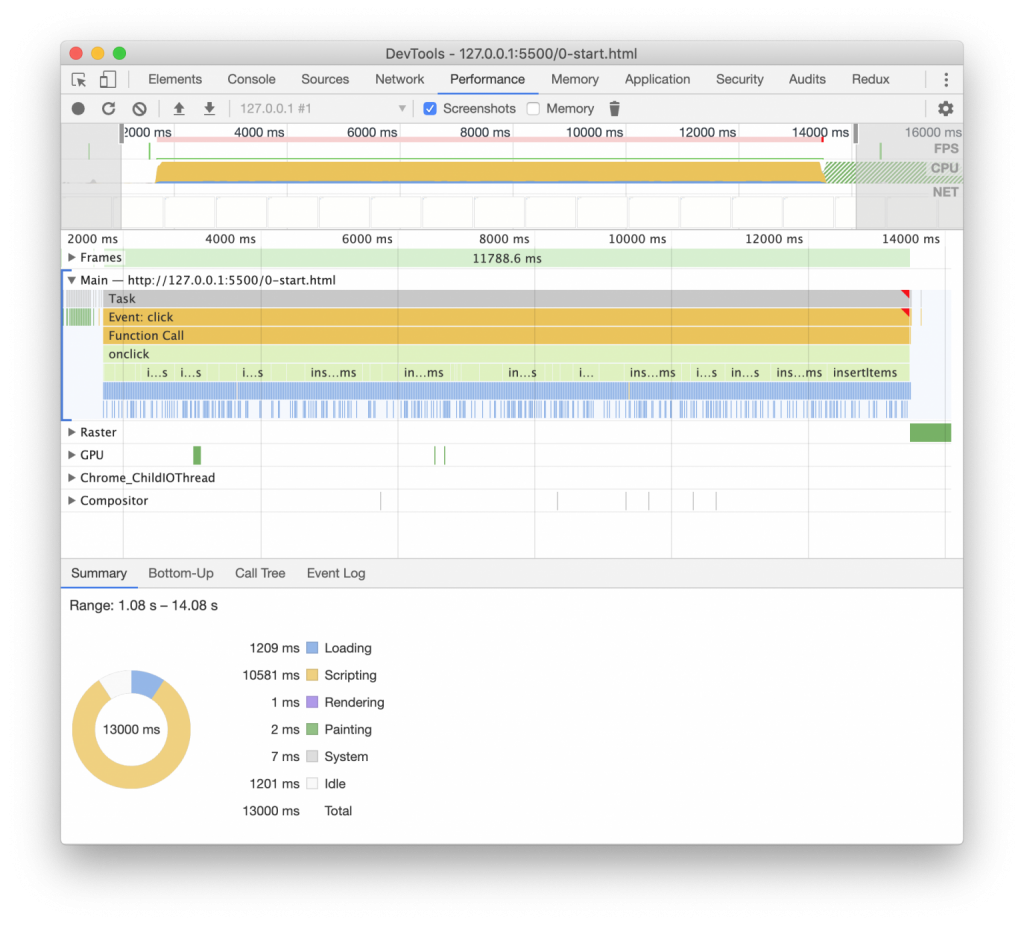
若是我們將上述程式中的第26行移除,則效能會改變如下圖所示:
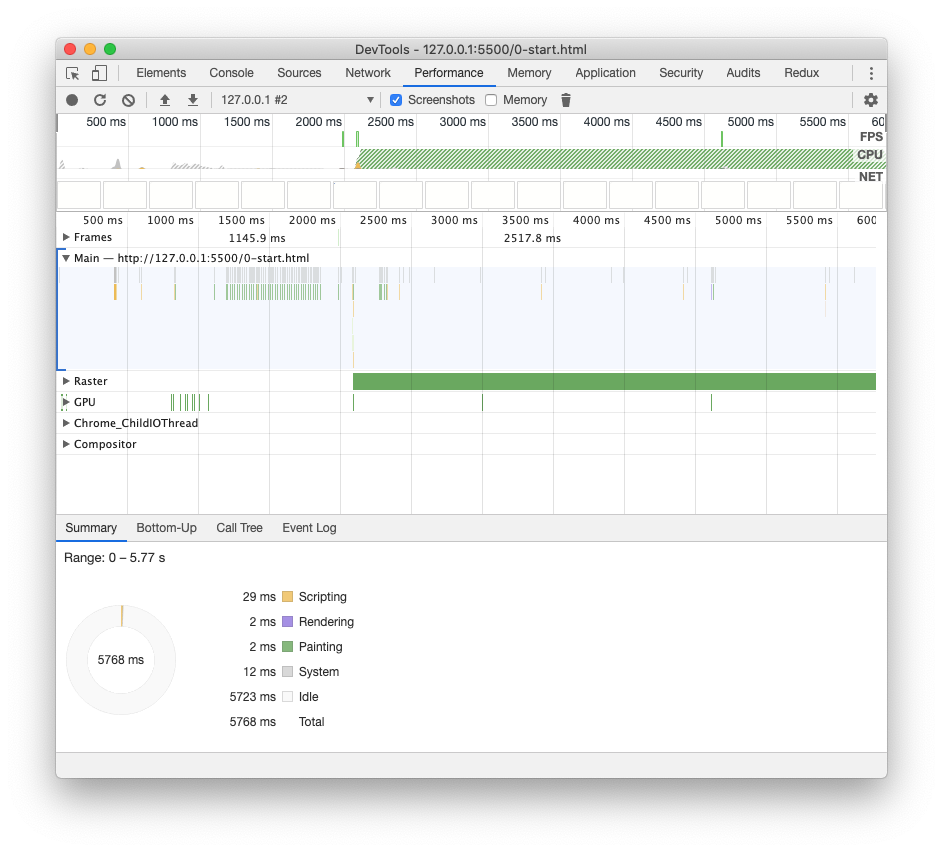
這樣可以很明確的了解效能殺手就是程式中的第26行,而這行程式的目的是去更新瀏覽器中的內容,若沒有這行沒辦法讓使用者看到最終的結果,因為我們必須透過這樣的方式更新DOM內容。
虛擬DOM的效能測試
同樣頁面的效果,我們在Vue裡面的作法如下:
<!DOCTYPE html> <html lang="zh"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title></title> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div id="app"> <ul> <li v-for="item in items">{{ item.message }}</li> </ul> <button @click="insertItems">Go</button> </div> </body> </html> <script> var vueData = { items: [ { message: 'Item 1' } ] } var app = new Vue({ el: '#app', data: vueData, methods: { insertItems: function(){ for(var i = 1; i <= 100000; i ++){ vueData.items[0].message = "Item " + i; } } } }) </script>
同樣的結果在Vue會在Javascript和瀏覽器中加入一層Virturl DOM,待Virturl DOM更新完畢之後,在寫入瀏覽器中。
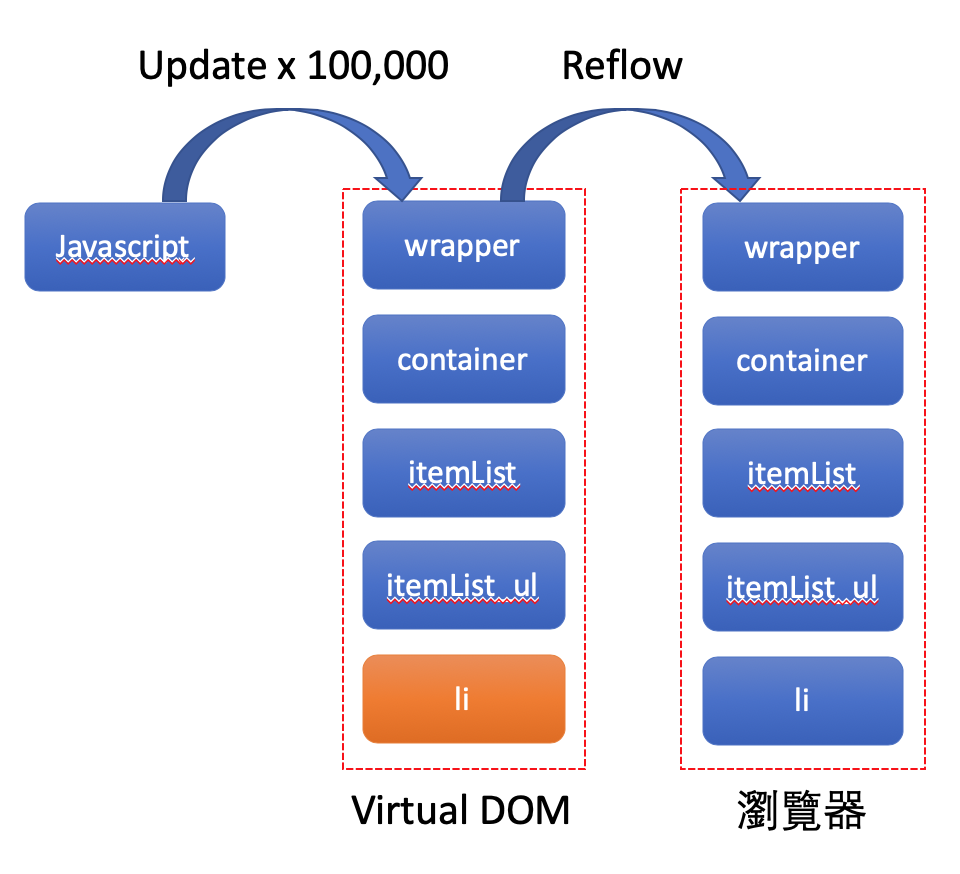
透過這樣的方法,使用這得到的一樣的效果,但大大提高了使用者端瀏覽器的效能,可以從下圖觀察的出來!
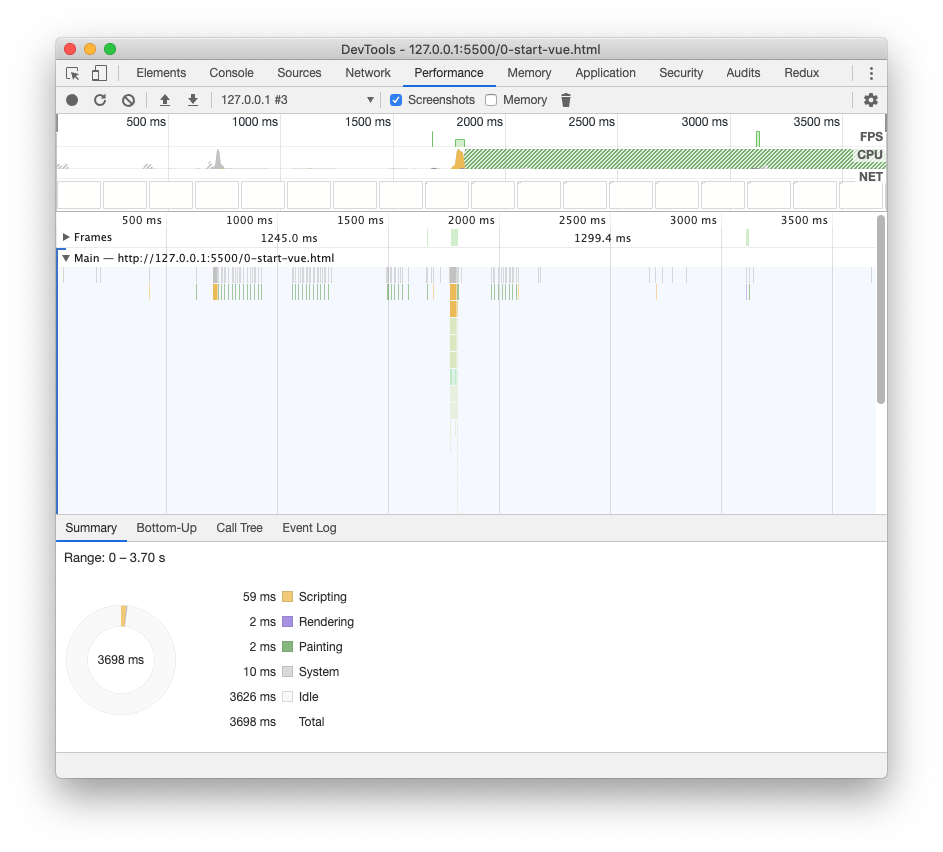
在Virtual DOM的架構中,會把程式的動作動作集中在Virtual DOM中運算,當確定整個頁面結構之後,再一次性地將結果繪製到頁面中,可以想像成原本的DOM操作就是在每一次在CPU運算之後,直接把結果寫到硬碟當中,而Virtual DOM就是在CPU與硬碟間加入了記憶體層,CPU運算後先將結果儲存在記憶體中,最後再將記憶體的資料一次性的寫入硬碟。
PS:記憶體的運算速度超過硬碟很多倍。
結論
綜合上述所說,網頁專案中採用前端框架,有著減少開發時間、易於維護、增加頁面效能…等優點,但若你的專案並不會大量與後端串接、製作上元件重複使用的機會不高、在頁面中也不太會對DOM進行Reflow與Repaint,可能是一個活動網頁、公司形象網頁…等,也許就沒有必要去選用一個前端框架,簡言之工具用在正確的地方,才能顯現出它的價值,當然目前符合使用框架的專案也一定非常多,也就是這樣的原因,才會造成前端框架的流行。
Добрый день!
Где купить диплом по актуальной специальности?
Мы можем предложить документы ВУЗов, расположенных на территории всей России. Можно купить качественно сделанный диплом от любого ВУЗа, за любой год, в том числе документы старого образца СССР. Дипломы выпускаются на “правильной” бумаге высшего качества. Это дает возможности делать настоящие дипломы, не отличимые от оригинала. Они заверяются необходимыми печатями и подписями.
Мы предлагаем дипломы любой профессии по приятным тарифам.
ast-diplomas.com/kupit-diplom-nizhnij-novgorod
Будем рады вам помочь!
buy atorvastatin online – zestril tablet bystolic 20mg pill
купить диплом рхту mandiplomik.ru .
речевое озвучивание речевое озвучивание .
Привет, друзья!
Наша компания предлагает заказать диплом в высоком качестве, который не отличить от оригинала без участия специалистов высокой квалификации со сложным оборудованием.
http://www.munhwahouse.or.kr/bbs/board.php?bo_table=free&wr_id=141231
Хорошей учебы!
Добрый день!
Наша компания предлагает выгодно заказать диплом, который выполнен на оригинальном бланке и заверен печатями, штампами, подписями должностных лиц. Документ пройдет любые проверки, даже с использованием специальных приборов. Решайте свои задачи быстро и просто с нашей компанией.
igia.cv.ua/index.php/forum/skrin-ka-propozitsij/1719-nado-bystro-zakazat-diplom-ob-obrazovanii#326243
Хорошей учебы!
Привет, друзья!
Где приобрести диплом по актуальной специальности?
Мы готовы предложить дипломы любых профессий по приятным ценам. Цена зависит от конкретной специальности, года получения и университета. Всегда стараемся поддерживать для заказчиков адекватную ценовую политику. Важно, чтобы документы были доступными для большого количества наших граждан.
Для вас можем предложить дипломы психологов, юристов, экономистов и любых других профессий по выгодным ценам.
chipokids.ru/company/personal/user/2309/
Всегда вам поможем!.
Привет, друзья!
Вопросы и ответы: можно ли быстро купить диплом старого образца?.
Приобрести диплом о высшем образовании.
famenest.com/read-blog/8813
The Allure regarding High-End Fashion
Style fans as well as high-end buyers frequently discover themselves enthralled by a elegance and prestige of high-end fashion. Starting with an detailed features of an Hermes Birkin towards a legendary layout regarding a Chanel 2.55, those items symbolize more than just simply style—they represent a good certain standing and exclusiveness. However, not everyone may afford to spend lavishly upon these particular high-end products, that offers led to a growing pattern regarding fake bags. For many, those replicas provide a manner to take pleasure in an splendor of high-fashion designs devoid of busting an loan provider.
The increase in popularity of replica handbags provides opened up a amazing interesting discussion regarding style, ethics, plus personal style. In some sort of weblog post, most of us can investigate the different features regarding some sort of movement, supplying style fans along with one detailed handbook for imitation purses. Simply by an conclusion, you may have a knowledge for you to create informed choices that will align themselves together with your beliefs plus aesthetics.
Honorable and Legal Considerations concerning Fake Bags
As soon as it pertains to replica handbags, 1 of an most demanding concerns can be a honorable plus legitimate effects. An generation and purchase regarding imitation products tend to be illegal inside a lot of countries, while it infringes intellectual residential property privileges. This specific not just impacts a profits of high-end brands but additionally increases concerns regarding the situations beneath that these particular imitations usually are produced.
Styling and Integrating Imitation Bags
Integrating imitation purses into your your collection can be both trendy trendy also moral. A single method is to mix premium replicas with real items plus other sustainable fashion goods. This does not only improves a total look but also promotes advances an eco-friendly fashion trend.
When styling replica purses, think about your event setting & a outfit. A classic classic imitation purse could add a touch of sophistication to a a dressy ensemble, while a fashionable pattern may render a casual look trendier. Don’t become scared to play with diverse patterns & accoutrements to develop an original plus personalized style.
Furthermore, looking after for replica bags is key vital to preserve their look look & durability. Routine washing plus appropriate storing may aid maintain your your handbags seeming new & stylish for longer.
The significance Significance of educated Consumer Selections
In the current modern fashion scene, knowledgeable consumer selections are more more crucial crucial than. Although imitation bags give a budget-friendly substitute to designer pieces, it key to think about the moral and legitimate consequences. By staying conscious of where & how to you purchase imitations, one can can relish premium apparel while staying true to your values principles.
It is additionally crucial to recall that personal personal fashion is not determined by name labels or price tags. Whether or not you one opts to invest in real designer items or explore exploring the domain of imitation bags, the key is finding finding items that make one feel confident and trendy.
Wrap-Up
Imitation bags have found a distinct special place in the fashion style world, providing a cost-effective and reachable substitute for luxury designer pieces. Although they arrive with their own particular set of of principled
Related Fashion Topics
Investigating the realm of fashion doesn’t conclude with copy bags. Here are some additional connected topics that might intrigue you:
Sustainable Fashion
Dive into the importance of sustainability in the apparel industry. Understand about green substances, moral fabrication techniques, and how to create a green collection.
Vintage and Thrift Fashion
Explore the charm of retro and second-hand garments. Find out how to source quality vintage items, the advantages of pre-owned apparel, and tips for incorporating these pieces into your current collection.
Do-It-Yourself Fashion and Upcycling
Be imaginative with Homemade fashion initiatives and repurposing old apparel. Get useful tips on transforming your wardrobe by creating your own accents or modifying existing articles to give them a renewed life.
Style on a Shoestring
Discover how to remain trendy without surpassing the savings. Investigate tricks for securing excellent deals, purchasing shrewdly during sales, and get your style budget.
Compact Collections
Grasp the notion of a minimalist wardrobe—creating a collection of important, versatile articles that can be matched and matched to make numerous looks. Examine the benefits of this simple method and how to apply it.
Apparel Tendencies and Projecting
Keep in front of the trend by discovering future apparel trends and field forecasts. Learn about pioneering designers, pivotal apparel shows, and the most recent necessary items.
Apparel and Tech
Discover how innovation is changing the apparel field. Themes involve advanced textiles, online try-ons, style tools, and the impact of social networks on fashion advertising and customer actions.
Figure Positivity and Apparel
Examine the link between style and body acceptance. Understand about labels promoting all-encompassing measurements, the importance of representation in fashion media, and how to style for different shapes.
Style History
Take a exploration through the history of style. Discover various periods, famous fashion moments, and how past fashions continue to influence contemporary style.
Apparel Photos and Blogging
Dive into the universe of style photography and blogs. Get guidance on how to take stunning style shots, start your own fashion website, and grow your reach in the digital space.
dior b23 reps
Привет!
Где заказать диплом по нужной специальности?
Заказать диплом о высшем образовании.
enriquerp.listbb.ru/viewtopic.php?f=4&t=428
Добрый день!
Мы предлагаем приобрести диплом в отличном качестве, неотличимый от оригинального документа без использования дорогостоящего оборудования и опытного специалиста.
http://www.daongil.com/bbs/board.php?bo_table=free&wr_id=298085
Успешной учебы!
Здравствуйте!
Всё, что нужно знать о покупке аттестата о среднем образовании без рисков.
Купить диплом ВУЗа.
cryptobomb.space/blogs/31/%D0%A0%D0%B0%D1%81%D1%88%D0%B8%D1%80%D0%B5%D0%BD%D0%BD%D0%BE%D0%B5-%D0%BE%D0%BF%D0%B8%D1%81%D0%B0%D0%BD%D0%B8%D0%B5-%D0%B7%D0%B0%D0%BA%D0%B0%D0%B7%D0%B0-%D0%B4%D0%BE%D0%BA%D1%83%D0%BC%D0%B5%D0%BD%D1%82%D0%BE%D0%B2-%D0%B2-%D0%BE%D0%BD%D0%BB%D0%B0%D0%B9%D0%BD-%D0%BC%D0%B0%D0%B3%D0%B0%D0%B7%D0%B8%D0%BD%D0%B5
Здравствуйте!
Узнайте, как безопасно купить диплом о высшем образовании
vk.com/id734172901?w=wall734172901_627
Поможем вам всегда!.
магазин аккаунтов роблокс магазин аккаунтов роблокс .
Привет, друзья!
Заказать диплом ВУЗа
Мы можем предложить документы техникумов, которые находятся на территории всей России. Можно заказать диплом от любого заведения, за любой год, включая сюда документы старого образца СССР. Документы делаются на бумаге высшего качества. Это дает возможности делать государственные дипломы, которые невозможно отличить от оригиналов. Документы будут заверены необходимыми печатями и подписями.
http://www.marqueze.net/miembros/matthewmorgan/profile/classic/
Окажем помощь!.
buy gasex pills – purchase gasex without prescription purchase diabecon generic
Kylie Jenner https://kylie-cosmetics.kylie-jenner-ar.com is an American model, media personality, and businesswoman, born on August 10, 1997 in Los Angeles, California.
Hi, just wanted to say, I liked this post. It was practical.
Keep on posting!Discover the unique blend of Java Burn ingredients and their benefits.
Explore how these natural components work together to boost metabolism and aid in weight loss.
gasex cost – gasex cost diabecon online order
Здравствуйте!
Как правильно приобрести диплом колледжа или ПТУ в России, важные моменты
dom-nam.ru/index.php/forum/stroitelnye-kompanii/50337-kupit-diplom#81222
Будем рады вам помочь!.
Продажа новых автомобилей Hongqi
https://hongqi-krasnoyarsk.ru/hongqi-h5 в Красноярске у официального дилера Хончи. Весь модельный ряд, все комплектации, выгодные цены, кредит, лизинг, трейд-ин
Upcoming fantasy MOBA https://bladesofthevoid.com evolved by Web3. Gacha perks, AI and crafting in one swirling solution!
Привет, друзья!
Купить диплом о высшем образовании
Мы предлагаем документы техникумов, расположенных в любом регионе России. Вы можете приобрести диплом за любой год, указав подходящую специальность и хорошие оценки за все дисциплины. Документы делаются на бумаге самого высшего качества. Это позволяет делать настоящие дипломы, не отличимые от оригиналов. Документы будут заверены необходимыми печатями и подписями.
usame.life/read-blog/46080
Окажем помощь!.
Казахский национальный технический университет https://satbayev.university им. К.Сатпаева
An Overview of Dynamic Balancing: Key Concepts and Applications
What is Dynamic Balancing?
Dynamic balancing is the technique of distributing mass in a rotor to minimize vibration while it rotates. This is vital for high-speed rotating equipment such as fans, pumps, turbines, and other machinery, where uneven mass distribution leads to significant vibrations, reducing the equipment’s lifespan and efficiency.
Dynamic balancing includes measuring and adjusting the mass in two planes perpendicular to the axis of rotation. This technique ensures precise mass distribution, reducing vibration and improving the reliability and durability of the equipment.
What is a Practical Example of Dynamic Balancing?
A common example of dynamic balancing is automobile wheel balancing. During vehicle operation, particularly at high speeds, even a slight imbalance in the wheels can cause significant vibrations, impacting driving comfort and safety.
To resolve this issue, each wheel is dynamically balanced. This involves placing balancing weights at specific points on the rim to counteract imbalances and minimize vibrations. This process allows automobile wheels to rotate smoothly and without vibrations at any speed.
How Are Static and Dynamic Balancing Different?
Two main types of balancing exist: static and dynamic.
Static Balance
Static balancing involves balancing mass in one plane. This method eliminates imbalance when the rotor is stationary. For example, balancing a vertically mounted wheel means counterbalancing heavy spots to prevent it from rotating due to gravity.
Dynamic Balancing Method
Dynamic balancing, as stated earlier, involves balancing mass in two planes. This method is crucial for high-speed rotating equipment because an imbalance in one plane can be compensated by an imbalance in the other, requiring a comprehensive approach to achieve perfect balance.
Dynamic balancing is a more complicated and precise process than static balancing. It requires specialized equipment and software to measure vibrations and determine where mass needs to be added or removed to achieve the best results.
Wrap-Up
Dynamic balancing is essential for maintaining the high performance and longevity of rotating equipment. Proper balancing reduces vibrations, decreases wear and tear, and prevents breakdowns. Examples like automobile wheel balancing show the importance of this process in everyday life. Understanding the difference between static and dynamic balancing helps select the right method for specific applications, ensuring reliable and efficient machinery operation.
https://johsocial.com/story6855448/balanset-revolutionizing-dynamic-balancing
Very good article. I definitely appreciate this site. Thanks!
Great site! I recommend to everyone!web design in fort smith arkansas
Приветствую. Может кто знает, где найти разные блоги о недвижимости? Пока нашел вот
Приветствую. Может кто знает, где найти полезные статьи о недвижимости? Пока нашел вот
Всем привет! Может кто знает, где найти разные блоги о недвижимости? Сейчас читаю вот