vue.js 學習手冊 框架的選擇與導入
這篇文章是vue.js學習手冊的第一篇文章,也是我認為最難寫的一篇文章,就像vue.js提到的他是一個“漸進式”框架,在這篇文章也想要跟各位分享選擇框架的一些原則,讓大家可以“漸進式”的了解為什麼我們在網頁開發時需要選擇一個框架來幫助我們,在選擇框架之前我們要先弄清楚,框架究竟可以幫助我們在網頁開發上的哪些部分,如果這些部分跟你要開發的項目並不媒合,那奉勸你別把單純的事情搞複雜了,而且你可能會開始討厭學習框架,但若反之,你一定會愛上框架,甚至覺得他讓你事半功倍。
強大的前、後端串接功能
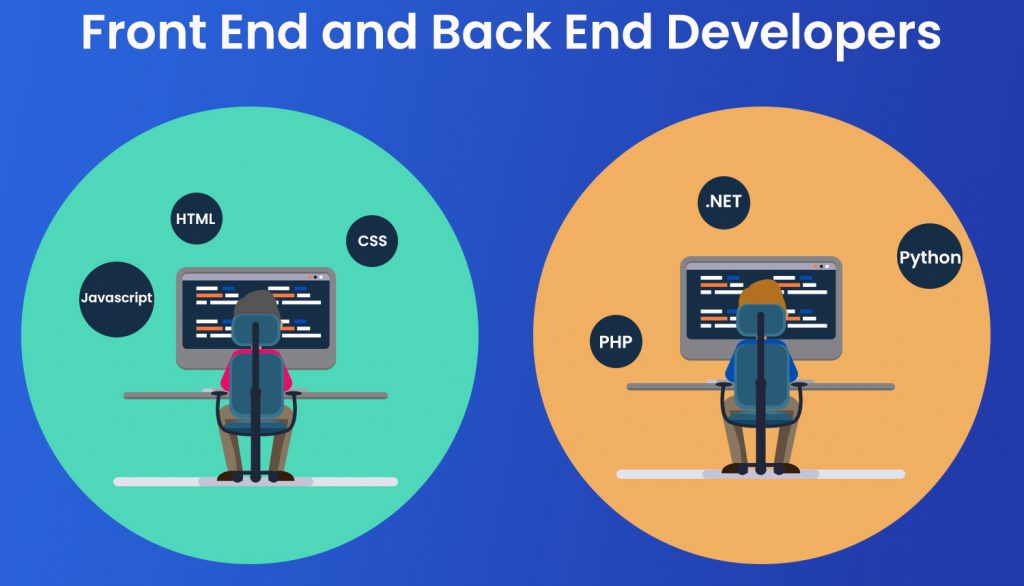
現代的網頁被要求除了有著摩登的前端UI之外,在網頁中的資料有常需要配合“大數據”下的資料進行呈現,說白話一點也就是網頁上面呈現的資料並不是寫死在頁面中的,而是透過後端資料庫取出來的,舉凡會員登入的名稱、購物網站中的商品資訊、新聞網站中的新聞就連你現在看到的這篇文章,也都是存放於資料庫中,網頁去對資料庫進行讀取後顯示在介面上的。
當然除了對資料庫進行讀取之外,網頁也會對資料庫進行儲存的動作,舉凡會員資料修改、商品訂單建立、網站偏好設定…等等,而框架在這方面有許多很好的方法,讓我們可以更周全快速的處理這方面的動作,節省許多開發的時間與減少Bug上的產生。
模組化開發架構
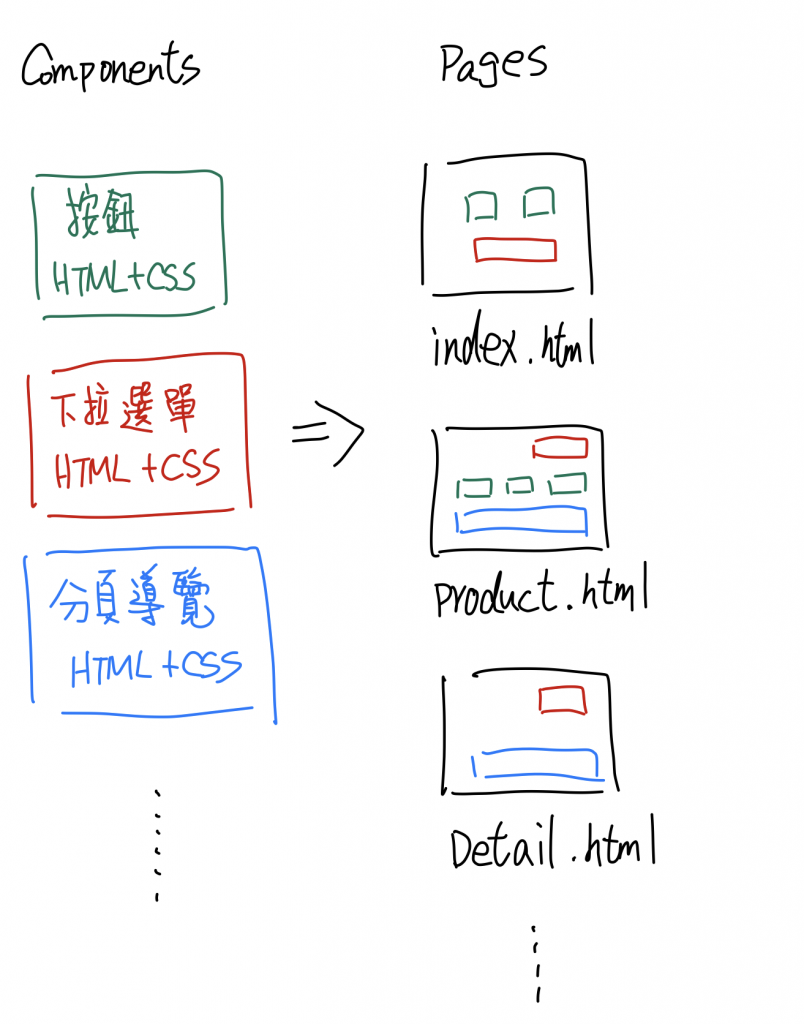
在一個大型網站中,可能有許多網頁中會出現相同風格的元素,例如:下拉式選單、按鈕、分頁導覽,是每一個頁面都會重複應用到的一些元件,傳統的網頁開發上就是在每一頁嵌入對應的HTML Code,這樣的做法非但不易維護,也會增加許多冗長且重複的程式碼。
模組化開發可以如上圖所示,將頁面中需重用的元素拉出來設計成一個Component,在不同頁面可以透過引入的方式置入該Component,而Component的維護可以統一在該Component中進行,可以減少大量維護上的時間。
透過 Virtual DOM 來提升頁面效能
現代的網頁前端框架為了提升頁面操作的效能都提供了Virtual DOM,在Vue.js 2.0中也引入Virtual DOM,比Vue.js 1.0的初始渲染速度提升了2~4倍,並大大降低了內存消耗,至於為何Virtual DOM能提昇網頁的效能,大家就必須了解我們透過Javascirpt更新實體DOM時會產生的效能問題開始了解。
實體DOM更新的效能測試
這邊製作一個簡單的範例對實體DOM和虛擬DOM的效能進行說明:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title></title> </head> <body> <div class="wrapper"> <div class="container"> <div class="itemList"> <ul id="itemList__ul"> <li id="liID">Item 1</li> </ul> </div> </div> <button onClick="insertItems()">Go</button> </div> </body> </html> <script> var itemData = ""; function insertItems() { for (var i = 1; i <= 100000; i++) { itemData = "Item " + i document.getElementById("liID").innerHTML = itemData; } } </script>
在HTML DOM的操作上,只要頁面元素有變更,就可能會觸發Reflow或Repaint這樣的動作,瀏覽器也會耗費相當多的資源在進行這些動作,以上述的例子來看,當我們按下頁面上的按鈕之後,就會透過迴圈去改變li的內容,這樣將會觸發多次的瀏覽器動作。
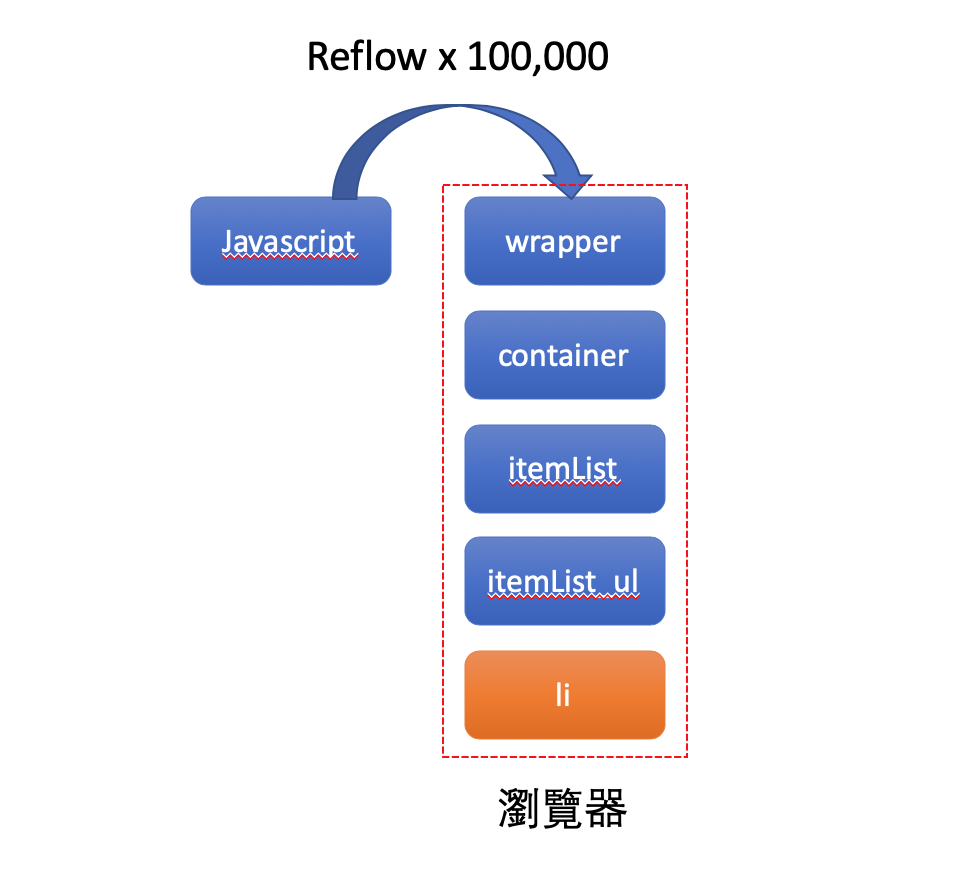
下圖是我們在Chrome中獲得的效能資訊:
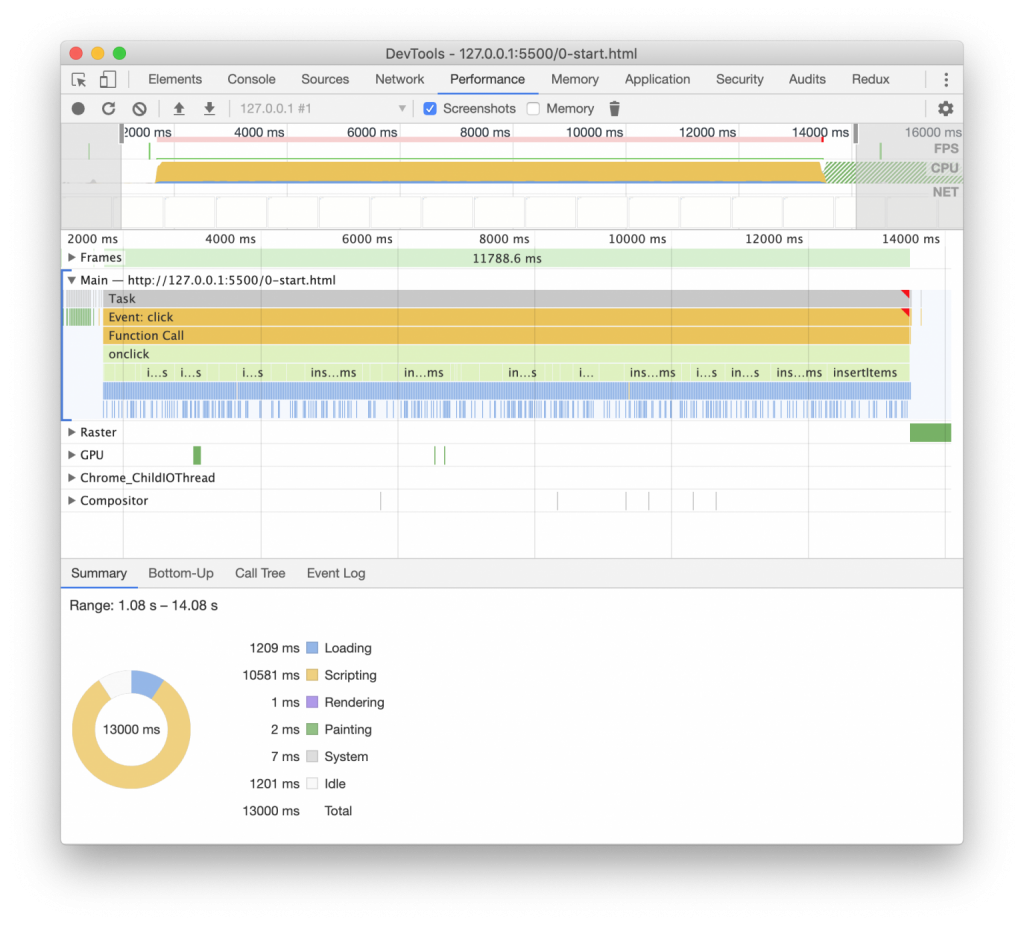
若是我們將上述程式中的第26行移除,則效能會改變如下圖所示:
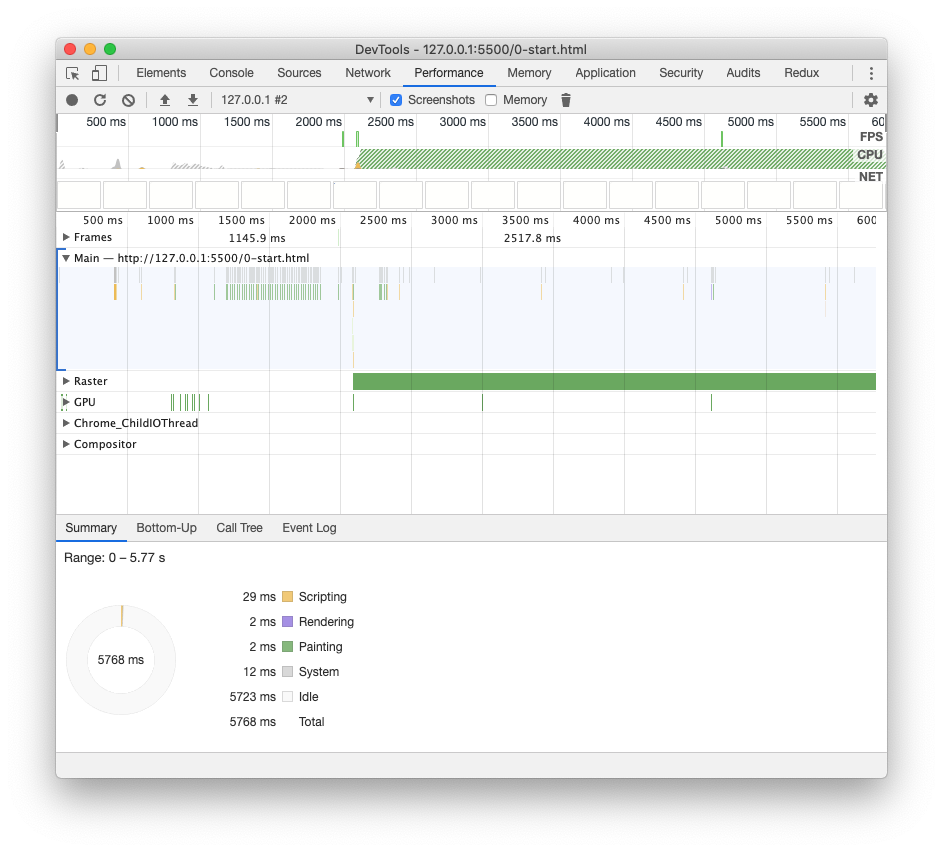
這樣可以很明確的了解效能殺手就是程式中的第26行,而這行程式的目的是去更新瀏覽器中的內容,若沒有這行沒辦法讓使用者看到最終的結果,因為我們必須透過這樣的方式更新DOM內容。
虛擬DOM的效能測試
同樣頁面的效果,我們在Vue裡面的作法如下:
<!DOCTYPE html> <html lang="zh"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title></title> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div id="app"> <ul> <li v-for="item in items">{{ item.message }}</li> </ul> <button @click="insertItems">Go</button> </div> </body> </html> <script> var vueData = { items: [ { message: 'Item 1' } ] } var app = new Vue({ el: '#app', data: vueData, methods: { insertItems: function(){ for(var i = 1; i <= 100000; i ++){ vueData.items[0].message = "Item " + i; } } } }) </script>
同樣的結果在Vue會在Javascript和瀏覽器中加入一層Virturl DOM,待Virturl DOM更新完畢之後,在寫入瀏覽器中。
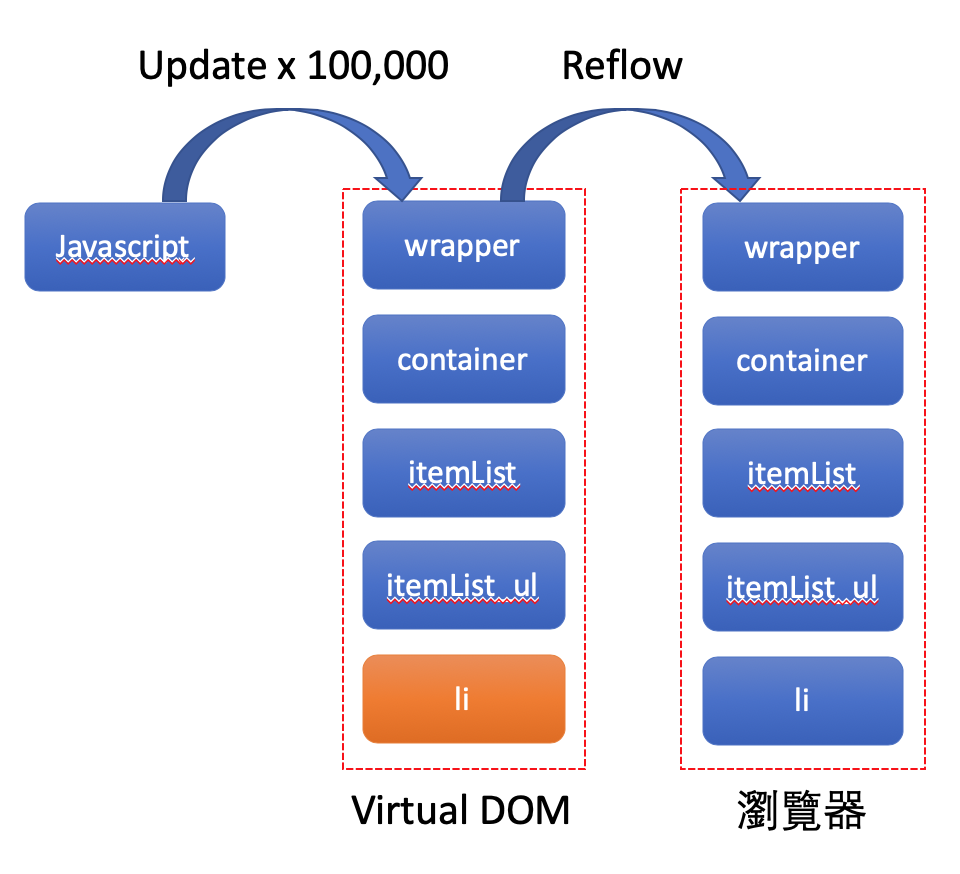
透過這樣的方法,使用這得到的一樣的效果,但大大提高了使用者端瀏覽器的效能,可以從下圖觀察的出來!
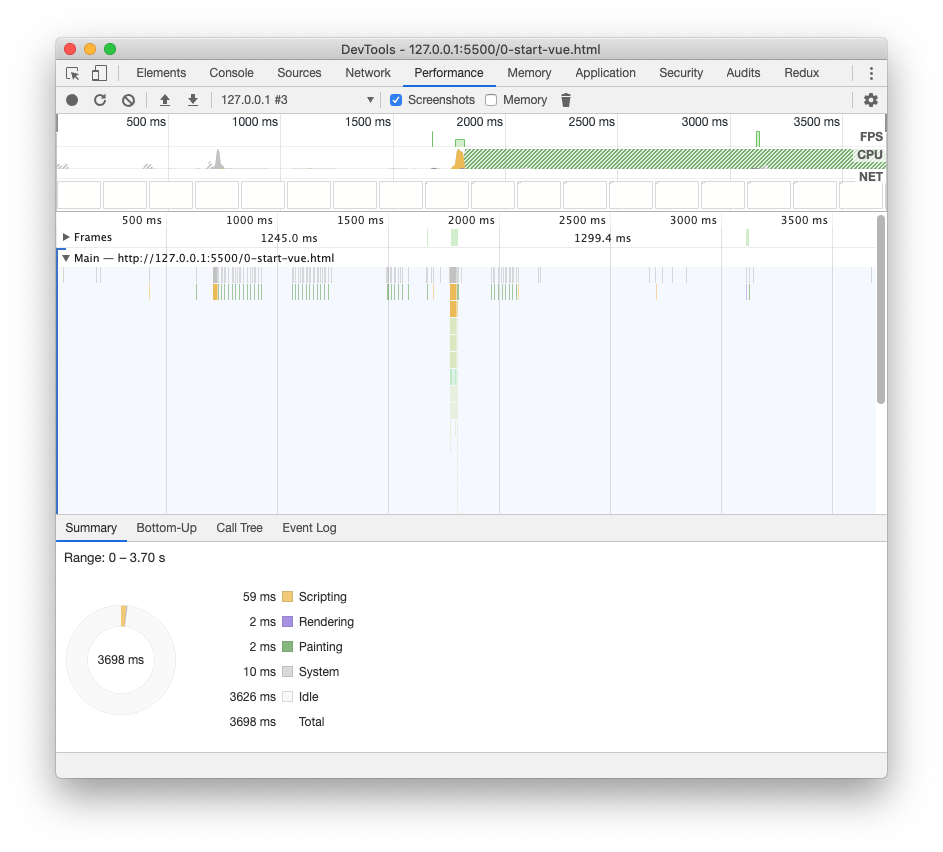
在Virtual DOM的架構中,會把程式的動作動作集中在Virtual DOM中運算,當確定整個頁面結構之後,再一次性地將結果繪製到頁面中,可以想像成原本的DOM操作就是在每一次在CPU運算之後,直接把結果寫到硬碟當中,而Virtual DOM就是在CPU與硬碟間加入了記憶體層,CPU運算後先將結果儲存在記憶體中,最後再將記憶體的資料一次性的寫入硬碟。
PS:記憶體的運算速度超過硬碟很多倍。
結論
綜合上述所說,網頁專案中採用前端框架,有著減少開發時間、易於維護、增加頁面效能…等優點,但若你的專案並不會大量與後端串接、製作上元件重複使用的機會不高、在頁面中也不太會對DOM進行Reflow與Repaint,可能是一個活動網頁、公司形象網頁…等,也許就沒有必要去選用一個前端框架,簡言之工具用在正確的地方,才能顯現出它的價值,當然目前符合使用框架的專案也一定非常多,也就是這樣的原因,才會造成前端框架的流行。
переговорные москва https://www.oborudovanie-peregovornyh-komnat.ru .
музыкальное оборудование для актового зала музыкальное оборудование для актового зала .
оборудование актового зала оборудование актового зала .
mexican drugstore online: medication from mexico pharmacy – best online pharmacies in mexico
Оборудование актовых залов http://oborudovanie-aktovogo-zala.ru/ .
medicine in mexico pharmacies: mexican mail order pharmacies – reputable mexican pharmacies online
световое оборудование для актового зала световое оборудование для актового зала .
оборудование для переговорных комнат оборудование для переговорных комнат .
Welcome to the World of Imitation Handbags
This allure of luxury bags is undeniable. Ranging from the iconic Louis Vuitton monogram to the timeless elegance of a Chanel flap purse, these items represent status and fashion. However, the expensive price labels often put them out of access for numerous fans. Welcome replica bags, a growing market that offers the look and feel of high-end brands at a fraction of the cost. But, are they valuable it? In this blog post, we will investigate the increasing trend of replica handbags, the moral issues involved, and provide practical tips for making knowledgeable choices.
Why Is Imitation Handbags Increasing Popularity?
The surge in the popularity of imitation bags can be credited to multiple factors. Firstly and foremost is affordability. Original designer bags frequently cost numerous of bucks, making them reachable only to a select few. Imitations offer a more budget-friendly option without sacrificing on style. Additionally, social media influences and famous endorsements have contributed to the need for these look-alike accessories. People desire to emulate their beloved stars without shattering the budget.
Another reason is the vast variety available in the imitation market. If you’re looking for a Gucci tote or a Prada clutch, chances are there’s a copy that suits your requirements. This wide array allows style aficionados to experiment with different styles without making a significant financial investment.
Nonetheless, it’s essential to think about the ethical implications of purchasing replicas. Although they provide an affordable way to stay trendy, they also raise questions about creative property rights and fair trade practices. Grasping these issues will help you make a more educated choice.
This Advantages and Cons of Purchasing Imitation Bags
Financial Benefits
This most clear benefit of buying a replica bag is the price savings. High-quality copies can look remarkably similar to their genuine counterparts, allowing you to enjoy the style without the exorbitant price tag. This financial advantage makes it easier for fashion lovers to diversify their collection.
Variety and Accessibility
Copies provide an chance to have various designs and designs that may elsewise be unattainable. This accessibility is particularly attractive to young workers and students who are still building their careers and can’t pay for to spend on luxury items.
Possible Law Concerns
However the downside, purchasing and possessing replica handbags can come with lawful dangers. In many nations, it is against the law to trade counterfeit products, and buyers can also face consequences. It’s crucial to be cognizant of the laws in your region to steer clear of any law-related issues.
Standard Issues
Although some copies are nearly impossible to tell apart from the genuine thing, others are badly made and won’t endure regular use. Low-quality materials and shoddy craftsmanship can result in a product that looks low-quality and breaks apart quickly.
Moral Considerations
Buying replicas also brings up ethical questions. By supporting the counterfeit market, buyers unintentionally contribute to unfair labor practices and the stealing of creative property. It’s essential to weigh these factors when determining whether to purchase a copy handbag.
How to Detect a Top-Notch Imitation
If you decide to go the imitation route, knowing how to recognize a premium goods is essential. Here’s what to gaze for:
Materials
The substances used in a handbag are a significant signal of its standard. Genuine skin is smooth and supple, while fake skin often seems rigid and artificial. Pay attention to the stitching as well; irregular or loose threads are a red flag.
Craftsmanship
Scrutinize the construction closely. High-quality imitations will have accurate, even sewing and neat edges. The fittings, such as zippers and buckles, should also be sturdy and free from stains or corrosion.
Brand Verification Techniques
Many luxury brands have specific features that are hard to imitate correctly. For example, Louis Vuitton handbags often have a distinctive serial number and exact patterns in the monogram that match perfectly. Getting to know yourself with these aspects can help you spot a real-looking replica.
An Moral Discussion About Copy Handbags
Impact on the Apparel Field
Copy bags have a significant influence on the style field. They undermine the significance of authentic designer items and can harm the brand’s standing. Designers invest heavily in creating one-of-a-kind, top-notch products, and counterfeits dilute this effort.
Customer Actions
The accessibility of replicas also shapes buyer conduct. Some assert that it broadens fashion by making luxury styles attainable to everyone. Others argue that it encourages a culture of throwaway, where people are more apt to acquire and toss items often.
Creative Trademark Laws
Supporting the replica market can be seen as approving the violation of intellectual property rights. Designers lose out on income and recognition when their creations are replicated and sold without permission.
Substitutes to Replica Bags
If you’re looking for budget-friendly ways to enjoy luxury fashion, explore these options:
Budget-Friendly Luxury Companies
Several brands provide high-quality, chic bags at a more accessible price point. Brands like Coach provide luxury styles without the large price tag.
Pre-Owned Platforms
Buying pre-owned designer bags is another excellent alternative. Websites like Poshmark sell certified pre-owned luxury products at a fraction of their initial cost. This strategy also promotes sustainability by offering items a fresh life.
Luxury Cooperations
Many designer brands collaborate with more budget-friendly brands to create exclusive lines. These collaborations often lead in distinct, exclusive pieces that enable you to own a designer item without shelling out designer prices.
Conclusion
In closing, copy handbags offer an enticing blend of cost-effectiveness and fashion, making them a favored choice for countless fashion fans.
маленькая переговорная маленькая переговорная .
переговорные комнаты под ключ переговорные комнаты под ключ .
оборудование для сцены актового зала оборудование для сцены актового зала .
переговорные москва http://oborudovanie-peregovornyh-komnat.ru .
световое оборудование для актового зала световое оборудование для актового зала .
оборудование актового зала оборудование актового зала .
mexican mail order pharmacies: mexican mail order pharmacies – best online pharmacies in mexico
световое оборудование для актового зала световое оборудование для актового зала .
оборудование переговорных комнат оборудование переговорных комнат .
best online pharmacies in mexico mexico drug stores pharmacies mexico pharmacies prescription drugs
переговорные комнаты переговорные комнаты .
mexican rx online mexico pharmacies prescription drugs п»їbest mexican online pharmacies
световое оборудование для актового зала световое оборудование для актового зала .
mexican rx online: mexican rx online – reputable mexican pharmacies online
акустическое оборудование для актового зала акустическое оборудование для актового зала .
ключ от комнаты совещаний ключ от комнаты совещаний .
переговорная комната под ключ переговорная комната под ключ .
оборудование для сцены актового зала оборудование для сцены актового зала .
оснащение переговорных комнат оснащение переговорных комнат .
оборудование для сцены актового зала оборудование для сцены актового зала .
маленькая переговорная маленькая переговорная .