vue.js 學習手冊 框架的選擇與導入
這篇文章是vue.js學習手冊的第一篇文章,也是我認為最難寫的一篇文章,就像vue.js提到的他是一個“漸進式”框架,在這篇文章也想要跟各位分享選擇框架的一些原則,讓大家可以“漸進式”的了解為什麼我們在網頁開發時需要選擇一個框架來幫助我們,在選擇框架之前我們要先弄清楚,框架究竟可以幫助我們在網頁開發上的哪些部分,如果這些部分跟你要開發的項目並不媒合,那奉勸你別把單純的事情搞複雜了,而且你可能會開始討厭學習框架,但若反之,你一定會愛上框架,甚至覺得他讓你事半功倍。
強大的前、後端串接功能
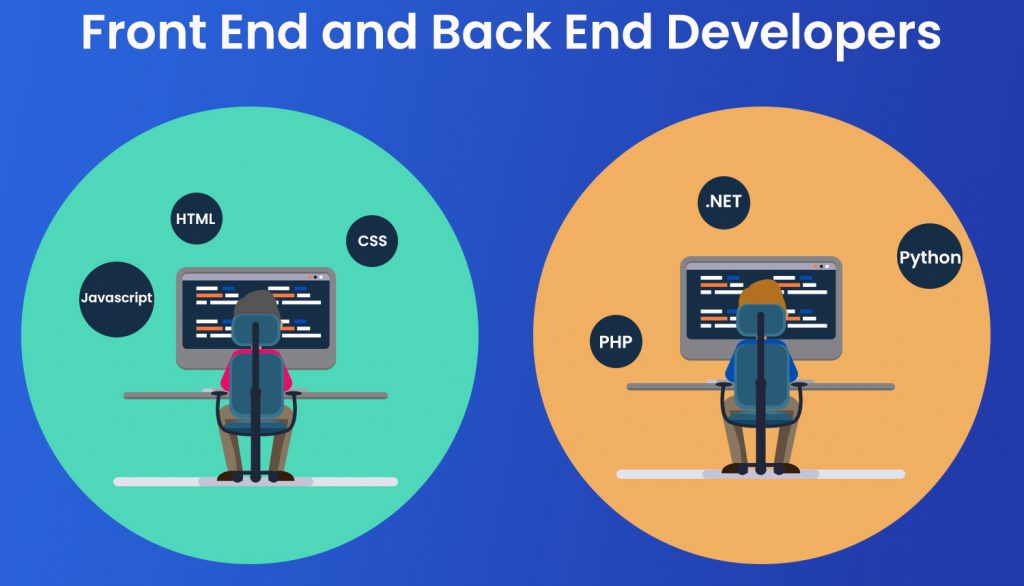
現代的網頁被要求除了有著摩登的前端UI之外,在網頁中的資料有常需要配合“大數據”下的資料進行呈現,說白話一點也就是網頁上面呈現的資料並不是寫死在頁面中的,而是透過後端資料庫取出來的,舉凡會員登入的名稱、購物網站中的商品資訊、新聞網站中的新聞就連你現在看到的這篇文章,也都是存放於資料庫中,網頁去對資料庫進行讀取後顯示在介面上的。
當然除了對資料庫進行讀取之外,網頁也會對資料庫進行儲存的動作,舉凡會員資料修改、商品訂單建立、網站偏好設定…等等,而框架在這方面有許多很好的方法,讓我們可以更周全快速的處理這方面的動作,節省許多開發的時間與減少Bug上的產生。
模組化開發架構
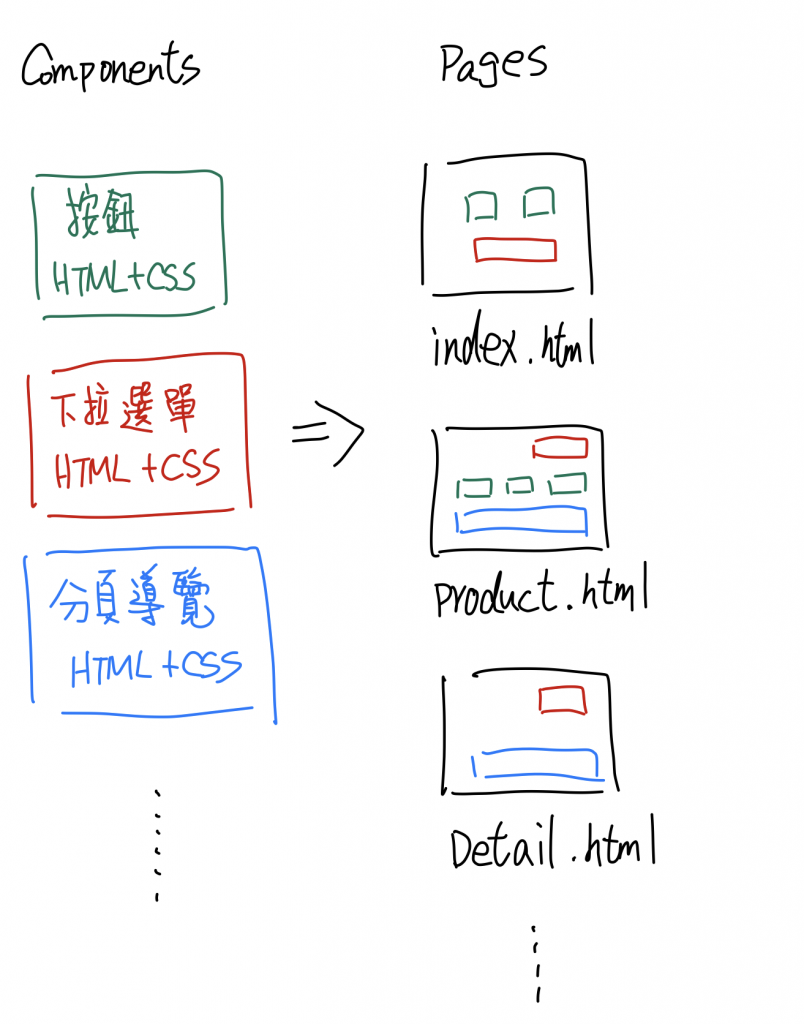
在一個大型網站中,可能有許多網頁中會出現相同風格的元素,例如:下拉式選單、按鈕、分頁導覽,是每一個頁面都會重複應用到的一些元件,傳統的網頁開發上就是在每一頁嵌入對應的HTML Code,這樣的做法非但不易維護,也會增加許多冗長且重複的程式碼。
模組化開發可以如上圖所示,將頁面中需重用的元素拉出來設計成一個Component,在不同頁面可以透過引入的方式置入該Component,而Component的維護可以統一在該Component中進行,可以減少大量維護上的時間。
透過 Virtual DOM 來提升頁面效能
現代的網頁前端框架為了提升頁面操作的效能都提供了Virtual DOM,在Vue.js 2.0中也引入Virtual DOM,比Vue.js 1.0的初始渲染速度提升了2~4倍,並大大降低了內存消耗,至於為何Virtual DOM能提昇網頁的效能,大家就必須了解我們透過Javascirpt更新實體DOM時會產生的效能問題開始了解。
實體DOM更新的效能測試
這邊製作一個簡單的範例對實體DOM和虛擬DOM的效能進行說明:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title></title> </head> <body> <div class="wrapper"> <div class="container"> <div class="itemList"> <ul id="itemList__ul"> <li id="liID">Item 1</li> </ul> </div> </div> <button onClick="insertItems()">Go</button> </div> </body> </html> <script> var itemData = ""; function insertItems() { for (var i = 1; i <= 100000; i++) { itemData = "Item " + i document.getElementById("liID").innerHTML = itemData; } } </script>
在HTML DOM的操作上,只要頁面元素有變更,就可能會觸發Reflow或Repaint這樣的動作,瀏覽器也會耗費相當多的資源在進行這些動作,以上述的例子來看,當我們按下頁面上的按鈕之後,就會透過迴圈去改變li的內容,這樣將會觸發多次的瀏覽器動作。
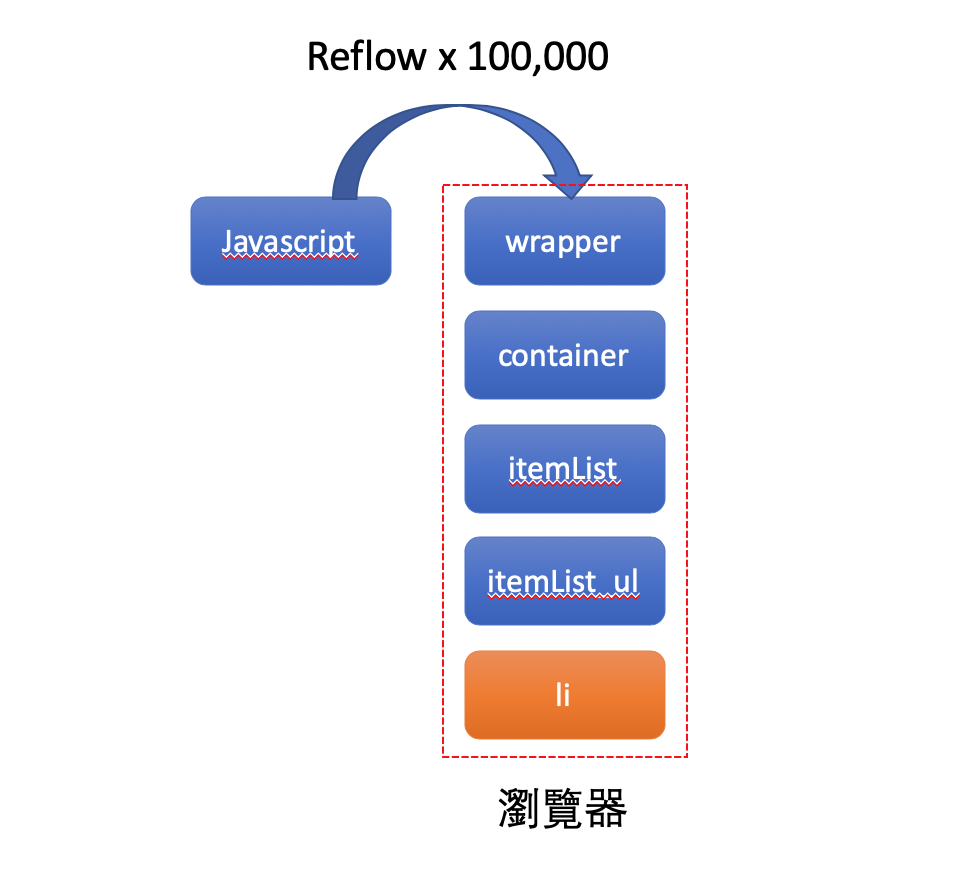
下圖是我們在Chrome中獲得的效能資訊:
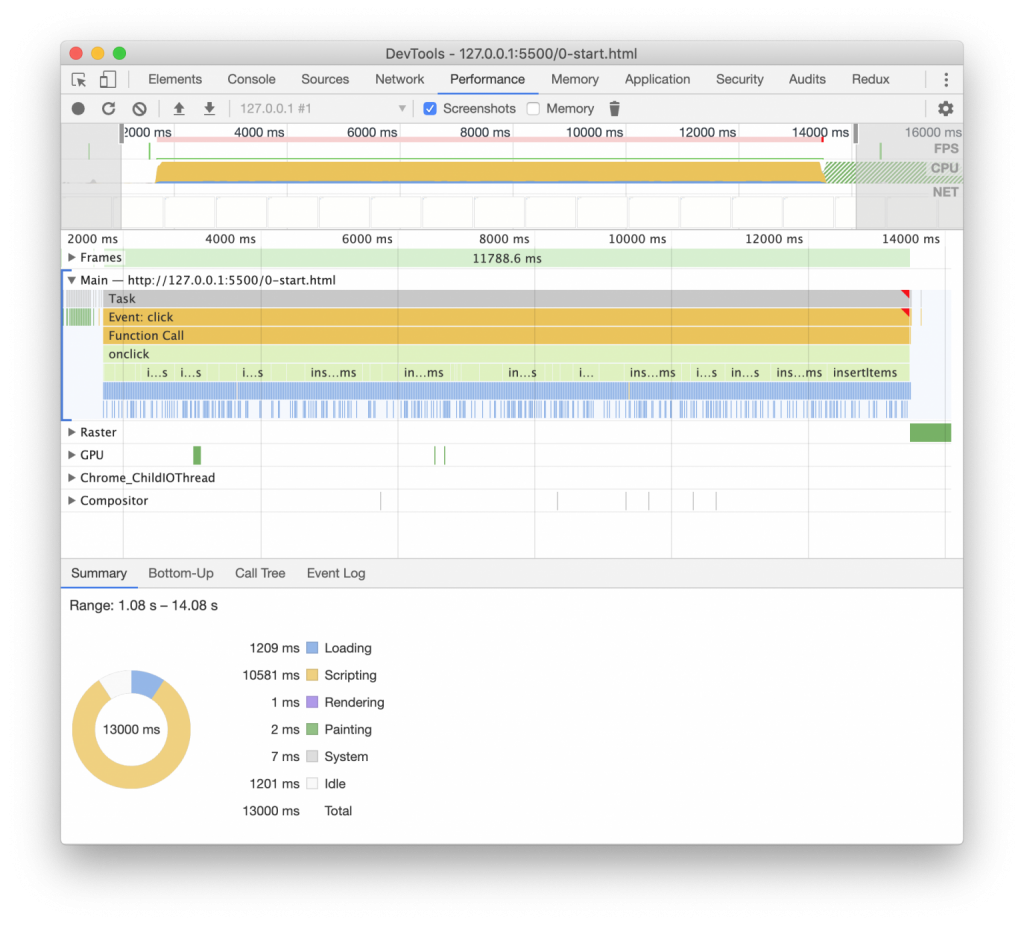
若是我們將上述程式中的第26行移除,則效能會改變如下圖所示:
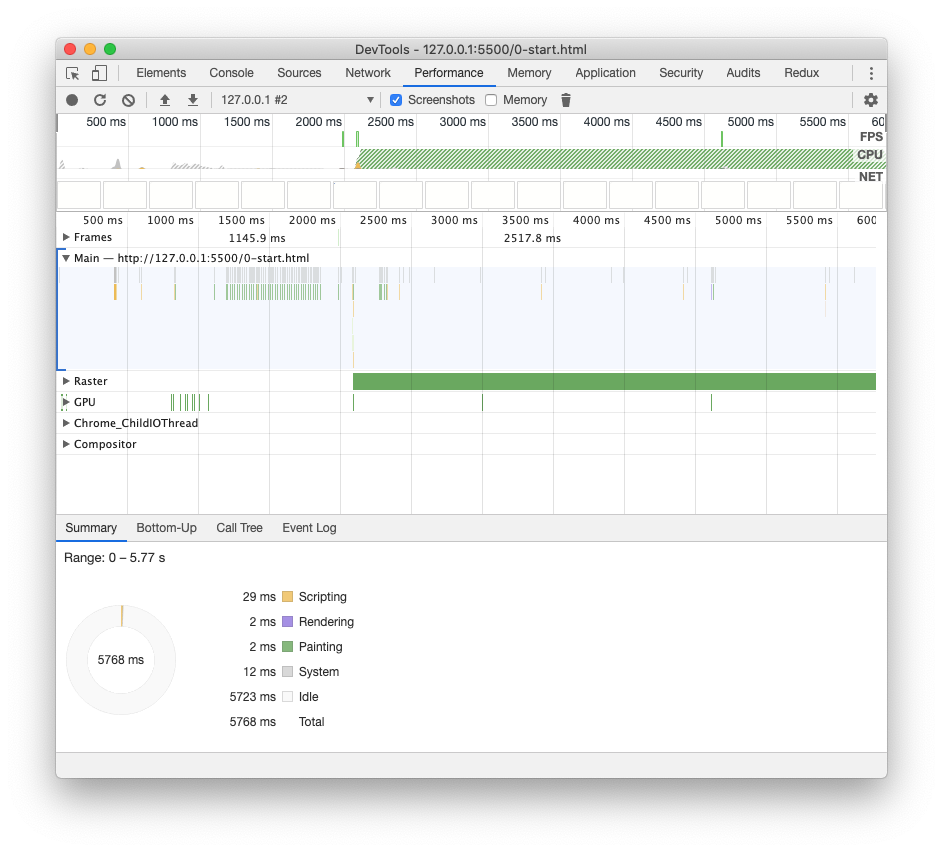
這樣可以很明確的了解效能殺手就是程式中的第26行,而這行程式的目的是去更新瀏覽器中的內容,若沒有這行沒辦法讓使用者看到最終的結果,因為我們必須透過這樣的方式更新DOM內容。
虛擬DOM的效能測試
同樣頁面的效果,我們在Vue裡面的作法如下:
<!DOCTYPE html> <html lang="zh"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title></title> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div id="app"> <ul> <li v-for="item in items">{{ item.message }}</li> </ul> <button @click="insertItems">Go</button> </div> </body> </html> <script> var vueData = { items: [ { message: 'Item 1' } ] } var app = new Vue({ el: '#app', data: vueData, methods: { insertItems: function(){ for(var i = 1; i <= 100000; i ++){ vueData.items[0].message = "Item " + i; } } } }) </script>
同樣的結果在Vue會在Javascript和瀏覽器中加入一層Virturl DOM,待Virturl DOM更新完畢之後,在寫入瀏覽器中。
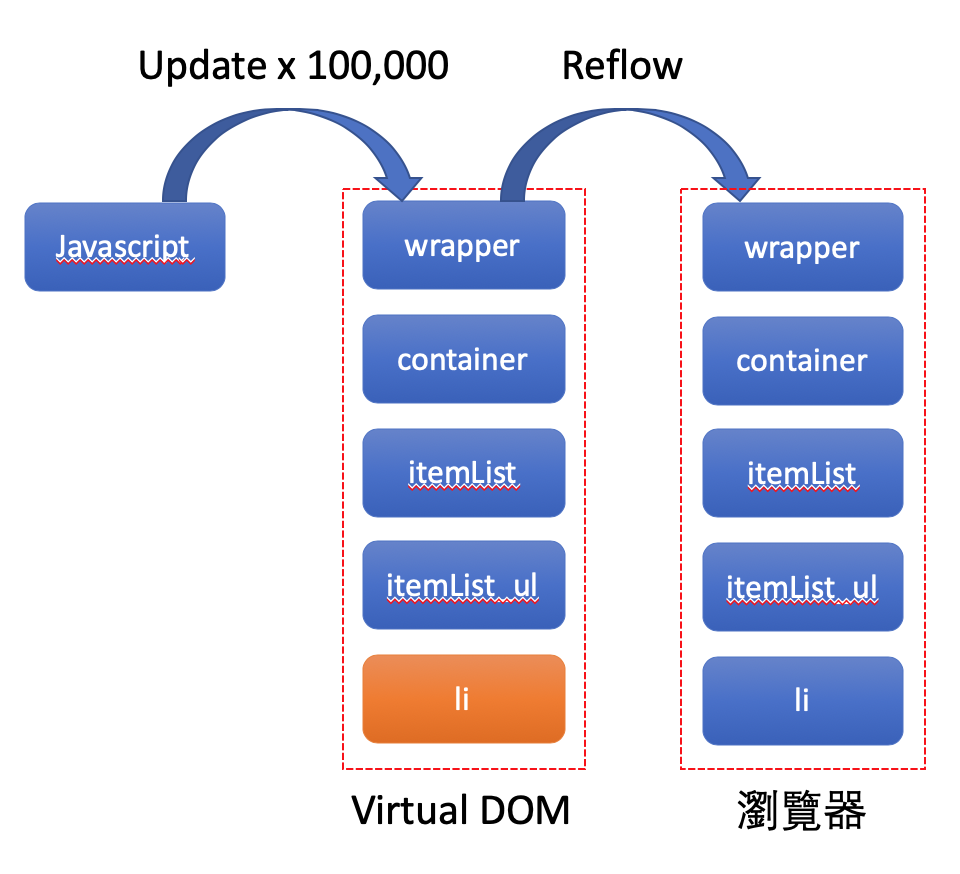
透過這樣的方法,使用這得到的一樣的效果,但大大提高了使用者端瀏覽器的效能,可以從下圖觀察的出來!
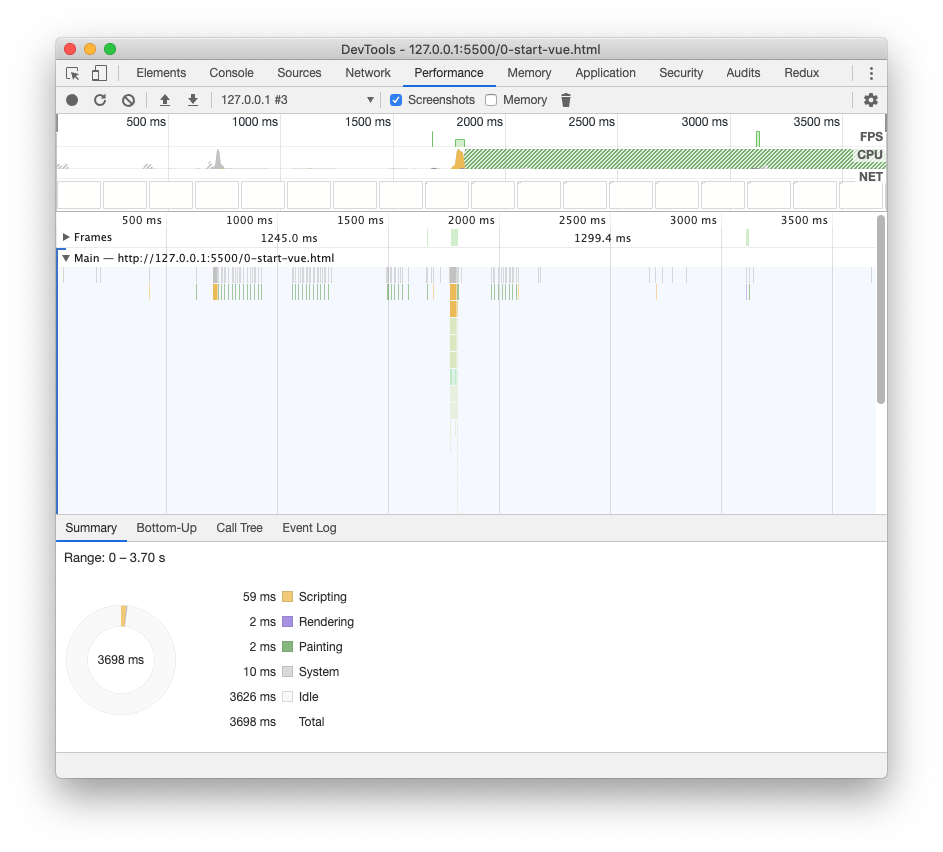
在Virtual DOM的架構中,會把程式的動作動作集中在Virtual DOM中運算,當確定整個頁面結構之後,再一次性地將結果繪製到頁面中,可以想像成原本的DOM操作就是在每一次在CPU運算之後,直接把結果寫到硬碟當中,而Virtual DOM就是在CPU與硬碟間加入了記憶體層,CPU運算後先將結果儲存在記憶體中,最後再將記憶體的資料一次性的寫入硬碟。
PS:記憶體的運算速度超過硬碟很多倍。
結論
綜合上述所說,網頁專案中採用前端框架,有著減少開發時間、易於維護、增加頁面效能…等優點,但若你的專案並不會大量與後端串接、製作上元件重複使用的機會不高、在頁面中也不太會對DOM進行Reflow與Repaint,可能是一個活動網頁、公司形象網頁…等,也許就沒有必要去選用一個前端框架,簡言之工具用在正確的地方,才能顯現出它的價值,當然目前符合使用框架的專案也一定非常多,也就是這樣的原因,才會造成前端框架的流行。
I have recently started a web site, thhe information you provide on this web sitge has helped me greatly.
Thank you for all of your time & work.
my blog: test nerves (Zachery)
ventolin diskus
We are happy to welcome you!
Sending newsletters via contact forms to the sites of firms via all countries of the world.
website =» contact-forms.su
WHAT IS THE MAILING OF SITES BY FEEDBACK FORMS?
This is a type of mailing using the contact forms that are located in the contact section of the website and filled by our programme in a preset mode with a rate of a few thousand contact forms per one minute, while the alphabetic and numeric code from the pictures (captcha) is also solved.
After that, the letters including your commercial offers are sent to the mailboxes of businesses.
When sending mailing by contact feedback forms, it turns out that each internet resource sends a message to its own e-mail and, therefore, all messages arrive in the inbox of the exact email in which the organisation is ready to receive the commercial information.
UTP:
Fast 100-percent informing of businesses and website owners about new commercial offers.
Search of new customers that other advertisements cannot find.
AIM:
Finding new customers that cannot be found through other advertisements.
Benefits:
1.one hundred % delivery of business letters.
2.Increase of the customer base.
3. Increase of the your market share.
4.Mailings based on topics and regionality.
5. Sending information to directors.
6. Supply/demand analysis.
7. Conducting marketing research.
8. Conducting surveys and studying public opinion.
9. Notification speed.
10. Cost.
11. Entering the international markets.
Our advantages:
1.When sending mailing by feedback forms, all letters arrive in the inbox folder. When sending bulk e-mails, this can reach up to 5 percent.
2.When sending mailing by contact forms it is possible to send a few million letters per 24 hours to inboxes. When sending e-newsletters a few thousands arrive in the inbox folders, all the rest often either are not delivered or arrive in the junk folder.
3. When sending mailing by feedback forms an e-mail does not get blocked by mails because it is sent from different websites. When sending bulk e-mails it is possible to send a few thousands of those but nevertheless all the IP addresses from which the mailing is sent will be banned.
4. When sending mailing by contact forms the minimum of macro-synonims is used to form headings and text of the letter. When sending e-mail newsletters, it is necessary to use synonyms («macros») for each phrase and create thousands different headings.
5. Many organisations try to hide their mailbox and only leave a contact form for contacting with them.
6. When sending e-newsletters, your cooperation offer can be delivered to every employee of the organisation, (which causes irritation) compared to mailing by contact forms where the information is received at the e-mail specifically set up for business offers.
7.Fifty percent of business e-mails are located on free mail systems, they are badly “searchable” by email, but when sending mailing by contact forms through these mail servers, all e-mails are one hundred percent delivered to the recipients.
8. Only 30%-40% of firms are published in the directories within several years, and all the rest are already placed in our VOIS databases and are waiting for your business offers.
Thus that emails of businesses from directories are full of spam, and therefore they will not have such outcome as when sending mailing by feedback forms using our the newest WHOIS databases.
9. Any kind of stop words in the headings or body of the letter can be sent through feedback forms. When sending e-newsletters, such messages either are not delivered the recipient or arrive in a spam folder.
The list of stop words of mail systems includes almost all phrases and words that encourage potential customers to take actions.
Application:
1. Increasing the customer base.
2. Quick informing of marketplaces about new business offers.
3. Accessing directors.
4.demand analysis.
5. Conducting tenders.
6.Conducting marketing research.
7.Studying public opinion and conducting surveys.
8. Searching for customers in other countries.
Reasons for purchasing this service:
1. one hundred percent delivery of your letters and commercial offers to millions of organisations all over the world.
Every website sends a e-mail to itself so all filters of mails are bypassed.
2.Mailing by feedback forms is an great way in in terms of conducting different marketing researches, surveys and studies of social opinion on any kind ofdirection and type of activity.
When sending mailing by contact forms, you will be sure that your message has been delivered to one hundred percent of users of your product and service and if a service or product is “badly promoted”, then the potential issue lies in in something else, for example in pricing.
At the same time, within seven days you will see real demand for your own services and products, you will not have to spend money on rent and other more expensive and time-consuming marketing activities.
3.Mailing by feedback forms is the quickest and the most economical way to get your service or product to the markets of other states.
4. Mailing by feedback forms is a tool for conducting all sorts of tenders.
5.Weekly update of the databases, as more than 150,000 new Internet resources, appear all over the world every day, and you, in turn, get new potential clients.
6. Full geographical scope for all states of the world.
7. We offer clients that are not available through other advertisement.
When sending mailing by feedback forms, you will be able to reach out that part of your potential clients, that is impossible to “break through” in automatic mode in any other way.
For instance, you will be able to send a business offer to those potential customers that were previously unavailable due to mail filters while sending bulk e-mails.
In the markets, there is a unusual situation: companies that got into the directories are actually filled with spam with all sorts of commercial offers while very little or no e-mails are sent to the remaining ones.
8. Unique technology of unraveling the captcha.
There are services for decoding numeric and alphabetic code (captcha/CAPTCHA). It costs a dollar to solve thousand CAPTCHAs.
It follows from this that, processing one million sites the robot unravels 1 million CAPTCHAs, which costs thousand $ only to decode captcha/CAPTCHA, and we provide this free of charge for you!
9. By ordering mailing by contact forms, you are promoting your service or product not to separate individuals, but to entire collectives, for instance domain .com, where more than one hundred and fifty million commercial organisations from all countries are accumulated (we have got samples of them from international zones for every country).
10. Mailing by contact forms also includes a subtype of text mailing
E-mail that is linked to the contact form is the primary electronic address of firms through which orders and cooperation offers are sent. This mail is also set up for phones as it is necessary to respond to the messages instantly so as not to lose the order or the relevance of the commercial offer.
11. The base of countries also includes all joint companies from all over the world working with or closely related to this country, for example, national communities and diasporas.
BAN, sanctions of search engines and mail systems?
These mailings are an alternative to sending mass e-mails, therefore search engine sanctions and “BAN” do not apply to them.
Mail delivers the data of messages to the inbox folder, as it passes through the “warm e-mail channel” from the new IP address of the website to the corporate e-mail of the same site.
In simple language, these mailings “live in emails” and e-mail filters do not react to them, because mail systems have great trust in communication channels between websites and corporate emails.
OUR DATABASES:
You can purchase our databases separately from the mailing by sending us a enquiry by contact form.
MORE THAN TWO THOUSAND VOIS DATABASES BY DOMAIN ZONES AND COUNTRIES OF THE WHOLE WORLD.
COLLECTIONS OF DATABASES BY THE MAIN CONTINENTS OF THE WORLD.
COLLECTIONS OF DATABASES BY THE MAIN LANGUAGES.
COLLECTIONS OF DATABASES BY THE MAIN CMS.
COLLECTIONS OF DATABASES BY THE MAIN COUNTRIES.
HOW TO MAKE AN ORDER FOR MAILING BY FEEDBACK FORMS:
The simplest text of the message + a few headings, the main goal is to interest the potential customer, and they will read the rest on your internet resource.
Most likely, all ads on your subject are already on the Internet, use the search bar and choose the most successful ones.
The headings are substituted randomly from .txt file.
Only text messages are sent, links are inserted without problems, they are all “clickable”. If the potential customer needs pictures or more detailed information, then you should forward the future customer to visit your internet resource.
In the letter:
Text without pictures, since pictures do not pass through the feedback form.
Your contact details:
Site address:
Fields to fill in:
Name:
Country:
City:
Site:
Several headings:
Electronic address for autoresponces:
website =» contact-forms.su
Price List =» contact-forms.su/en/price/
坐在家里赚点钱。 http://go.afalobo.com/0ide
https://zithromaxforsale.shop/# zithromax 500
сувенирная продукция с логотипом suvenirnaya-produkciya-s-logotipom.ru
best price for generic propecia
where to buy over the counter clomid clomid 100mg price in india
现在在网上赚钱更容易了。 http://go.afalobo.com/0ide
medication bactrim
赚钱可以非常容易,如果你使用这个机器人。 https://tam.borisvonsmercek.de/tam
generic amoxil 500 mg amoxicillin cost australia
compare prednisone prices prednisone online india
这个机器人可以给你带来24/7的钱。 https://tam.fannyberlin.se/tam
prednisone 10mg canada 40 mg prednisone pill
在线收入是你成功的关键。 https://tam.fannyberlin.se/tam
bupropion hcl 100mg
让你钱包变厚的最快方法就在这里。 https://collectif-hameb.fr/tam
cipro 250mg
plaquenil for sale online generic plaquenil 200 mg
nolvadex 10mg online india
Фильмы и Сериалы · Первый канал.
. Кино тор любовь и гром фильм 2022 анвап.
Фильмы. Смотреть фильмы онлайн.
zanaflex reviews fibromyalgia tizanidine buy does zanaflex cause dry mouth what is the recomended dosage of zanaflex for a 222 pound man
viagra cost per pill where to buy viagra online
推出最好的投资工具,今天开始赚钱。 https://baleti-design.fr/tam
gabapentin online
metformin 227
bupropion sr
I’ll immediately clutch your rss feed as I can’t in finding your e-mail subscription hyperlink or
e-newsletter service. Do you have any? Please let
me know in order that I maay subscribe. Thanks.
My webpage :: cpc exam (https://examenhub.com)
viagra price viagra